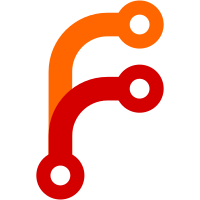
Adds validation for urls we use for our shapes and assets. This PR includes a migration so we should check that existing rooms still load correctly. There might be some that won't, but that means that they had invalid url set. ### Change Type - [x] `patch` — Bug fix - [ ] `minor` — New feature - [ ] `major` — Breaking change - [ ] `dependencies` — Changes to package dependencies[^1] - [ ] `documentation` — Changes to the documentation only[^2] - [ ] `tests` — Changes to any test code only[^2] - [ ] `internal` — Any other changes that don't affect the published package[^2] - [ ] I don't know [^1]: publishes a `patch` release, for devDependencies use `internal` [^2]: will not publish a new version ### Test Plan 1. Existing rooms should still load correctly (there should be no validation errors). 2. Adding new images and videos should also work (test both local and multiplayer rooms as they handle assets differently). - [x] Unit Tests - [ ] End to end tests ### Release Notes - Add validation to urls. --------- Co-authored-by: alex <alex@dytry.ch>
5.8 KiB
5.8 KiB
API Report File for "@tldraw/validate"
Do not edit this file. It is a report generated by API Extractor.
import { JsonValue } from '@tldraw/utils';
// @public
const any: Validator<any>;
// @public
const array: Validator<unknown[]>;
// @public
function arrayOf<T>(itemValidator: Validatable<T>): ArrayOfValidator<T>;
// @public (undocumented)
export class ArrayOfValidator<T> extends Validator<T[]> {
constructor(itemValidator: Validatable<T>);
// (undocumented)
readonly itemValidator: Validatable<T>;
// (undocumented)
lengthGreaterThan1(): Validator<T[]>;
// (undocumented)
nonEmpty(): Validator<T[]>;
}
// @public
const bigint: Validator<bigint>;
// @public
const boolean: Validator<boolean>;
// @public
function dict<Key extends string, Value>(keyValidator: Validatable<Key>, valueValidator: Validatable<Value>): DictValidator<Key, Value>;
// @public (undocumented)
export class DictValidator<Key extends string, Value> extends Validator<Record<Key, Value>> {
constructor(keyValidator: Validatable<Key>, valueValidator: Validatable<Value>);
// (undocumented)
readonly keyValidator: Validatable<Key>;
// (undocumented)
readonly valueValidator: Validatable<Value>;
}
// @public
const integer: Validator<number>;
// @public
function jsonDict(): DictValidator<string, JsonValue>;
// @public
const jsonValue: Validator<JsonValue>;
// @public
const linkUrl: Validator<string>;
// @public
function literal<T extends boolean | number | string>(expectedValue: T): Validator<T>;
// @public (undocumented)
function literalEnum<const Values extends readonly unknown[]>(...values: Values): Validator<Values[number]>;
// @public
function model<T extends {
readonly id: string;
}>(name: string, validator: Validatable<T>): Validator<T>;
// @public
const nonZeroInteger: Validator<number>;
// @public
const nonZeroNumber: Validator<number>;
// @public (undocumented)
function nullable<T>(validator: Validatable<T>): Validator<null | T>;
// @public
const number: Validator<number>;
// @public
function object<Shape extends object>(config: {
readonly [K in keyof Shape]: Validatable<Shape[K]>;
}): ObjectValidator<Shape>;
// @public (undocumented)
export class ObjectValidator<Shape extends object> extends Validator<Shape> {
constructor(config: {
readonly [K in keyof Shape]: Validatable<Shape[K]>;
}, shouldAllowUnknownProperties?: boolean);
// (undocumented)
allowUnknownProperties(): ObjectValidator<Shape>;
// (undocumented)
readonly config: {
readonly [K in keyof Shape]: Validatable<Shape[K]>;
};
extend<Extension extends Record<string, unknown>>(extension: {
readonly [K in keyof Extension]: Validatable<Extension[K]>;
}): ObjectValidator<Shape & Extension>;
}
// @public (undocumented)
function optional<T>(validator: Validatable<T>): Validator<T | undefined>;
// @public
const positiveInteger: Validator<number>;
// @public
const positiveNumber: Validator<number>;
// @public (undocumented)
function setEnum<T>(values: ReadonlySet<T>): Validator<T>;
// @public
const srcUrl: Validator<string>;
// @public
const string: Validator<string>;
declare namespace T {
export {
literal,
arrayOf,
object,
jsonDict,
dict,
union,
model,
setEnum,
optional,
nullable,
literalEnum,
ValidatorFn,
Validatable,
ValidationError,
TypeOf,
Validator,
ArrayOfValidator,
ObjectValidator,
UnionValidator,
DictValidator,
unknown,
any,
string,
number,
positiveNumber,
nonZeroNumber,
integer,
positiveInteger,
nonZeroInteger,
boolean,
bigint,
array,
unknownObject,
jsonValue,
linkUrl,
srcUrl
}
}
export { T }
// @public (undocumented)
type TypeOf<V extends Validatable<unknown>> = V extends Validatable<infer T> ? T : never;
// @public
function union<Key extends string, Config extends UnionValidatorConfig<Key, Config>>(key: Key, config: Config): UnionValidator<Key, Config>;
// @public (undocumented)
export class UnionValidator<Key extends string, Config extends UnionValidatorConfig<Key, Config>, UnknownValue = never> extends Validator<TypeOf<Config[keyof Config]> | UnknownValue> {
constructor(key: Key, config: Config, unknownValueValidation: (value: object, variant: string) => UnknownValue);
// (undocumented)
validateUnknownVariants<Unknown>(unknownValueValidation: (value: object, variant: string) => Unknown): UnionValidator<Key, Config, Unknown>;
}
// @public
const unknown: Validator<unknown>;
// @public (undocumented)
const unknownObject: Validator<Record<string, unknown>>;
// @public (undocumented)
type Validatable<T> = {
validate: (value: unknown) => T;
};
// @public (undocumented)
class ValidationError extends Error {
constructor(rawMessage: string, path?: ReadonlyArray<number | string>);
// (undocumented)
name: string;
// (undocumented)
readonly path: ReadonlyArray<number | string>;
// (undocumented)
readonly rawMessage: string;
}
// @public (undocumented)
export class Validator<T> implements Validatable<T> {
constructor(validationFn: ValidatorFn<T>);
check(name: string, checkFn: (value: T) => void): Validator<T>;
// (undocumented)
check(checkFn: (value: T) => void): Validator<T>;
isValid(value: unknown): value is T;
nullable(): Validator<null | T>;
optional(): Validator<T | undefined>;
refine<U>(otherValidationFn: (value: T) => U): Validator<U>;
validate(value: unknown): T;
// (undocumented)
readonly validationFn: ValidatorFn<T>;
}
// @public (undocumented)
type ValidatorFn<T> = (value: unknown) => T;
// (No @packageDocumentation comment for this package)