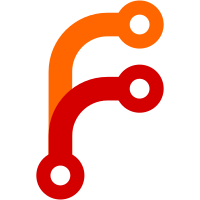
Typescript's type aliases (`type X = thing`) can refer to basically anything, which makes it hard to write an automatic document formatter for them. Interfaces on the other hand are only object, so they play much nicer with docs. Currently, object-flavoured type aliases don't really get expanded at all on our docs site, which means we have a bunch of docs content that's not shown on the site. This diff introduces a lint rule that forces `interface X {foo: bar}`s instead of `type X = {foo: bar}` where possible, as it results in a much better documentation experience: Before: <img width="437" alt="Screenshot 2024-05-22 at 15 24 13" src="https://github.com/tldraw/tldraw/assets/1489520/32606fd1-6832-4a1e-aa5f-f0534d160c92"> After: <img width="431" alt="Screenshot 2024-05-22 at 15 33 01" src="https://github.com/tldraw/tldraw/assets/1489520/4e0d59ee-c38e-4056-b9fd-6a7f15d28f0f"> ### Change Type - [x] `sdk` — Changes the tldraw SDK - [x] `docs` — Changes to the documentation, examples, or templates. - [x] `improvement` — Improving existing features
383 lines
11 KiB
Markdown
383 lines
11 KiB
Markdown
## API Report File for "@tldraw/utils"
|
|
|
|
> Do not edit this file. It is a report generated by [API Extractor](https://api-extractor.com/).
|
|
|
|
```ts
|
|
|
|
// @internal
|
|
export function annotateError(error: unknown, annotations: Partial<ErrorAnnotations>): void;
|
|
|
|
// @internal (undocumented)
|
|
export function areArraysShallowEqual<T>(arr1: readonly T[], arr2: readonly T[]): boolean;
|
|
|
|
// @internal (undocumented)
|
|
export function areObjectsShallowEqual<T extends Record<string, unknown>>(obj1: T, obj2: T): boolean;
|
|
|
|
// @internal (undocumented)
|
|
export const assert: (value: unknown, message?: string) => asserts value;
|
|
|
|
// @internal (undocumented)
|
|
export const assertExists: <T>(value: T, message?: string | undefined) => NonNullable<T>;
|
|
|
|
// @internal
|
|
export function clearLocalStorage(): void;
|
|
|
|
// @internal
|
|
export function clearSessionStorage(): void;
|
|
|
|
// @internal (undocumented)
|
|
export function compact<T>(arr: T[]): NonNullable<T>[];
|
|
|
|
// @public
|
|
export function debounce<T extends unknown[], U>(callback: (...args: T) => PromiseLike<U> | U, wait: number): {
|
|
(...args: T): Promise<U>;
|
|
cancel(): void;
|
|
};
|
|
|
|
// @public
|
|
export function dedupe<T>(input: T[], equals?: (a: any, b: any) => boolean): T[];
|
|
|
|
// @public (undocumented)
|
|
export const DEFAULT_SUPPORT_VIDEO_TYPES: readonly string[];
|
|
|
|
// @public (undocumented)
|
|
export const DEFAULT_SUPPORTED_IMAGE_TYPES: readonly string[];
|
|
|
|
// @public (undocumented)
|
|
export const DEFAULT_SUPPORTED_MEDIA_TYPE_LIST: string;
|
|
|
|
// @internal
|
|
export function deleteFromLocalStorage(key: string): void;
|
|
|
|
// @internal
|
|
export function deleteFromSessionStorage(key: string): void;
|
|
|
|
// @public (undocumented)
|
|
export interface ErrorResult<E> {
|
|
// (undocumented)
|
|
readonly error: E;
|
|
// (undocumented)
|
|
readonly ok: false;
|
|
}
|
|
|
|
// @internal (undocumented)
|
|
export function exhaustiveSwitchError(value: never, property?: string): never;
|
|
|
|
// @public (undocumented)
|
|
export type Expand<T> = T extends infer O ? {
|
|
[K in keyof O]: O[K];
|
|
} : never;
|
|
|
|
// @public
|
|
export class FileHelpers {
|
|
static blobToDataUrl(file: Blob): Promise<string>;
|
|
static blobToText(file: Blob): Promise<string>;
|
|
// (undocumented)
|
|
static dataUrlToArrayBuffer(dataURL: string): Promise<ArrayBuffer>;
|
|
}
|
|
|
|
// @internal
|
|
export function filterEntries<Key extends string, Value>(object: {
|
|
[K in Key]: Value;
|
|
}, predicate: (key: Key, value: Value) => boolean): {
|
|
[K in Key]: Value;
|
|
};
|
|
|
|
// @internal
|
|
export function fpsThrottle(fn: () => void): () => void;
|
|
|
|
// @internal (undocumented)
|
|
export function getErrorAnnotations(error: Error): ErrorAnnotations;
|
|
|
|
// @public
|
|
export function getFirstFromIterable<T = unknown>(set: Map<any, T> | Set<T>): T;
|
|
|
|
// @internal
|
|
export function getFromLocalStorage(key: string): null | string;
|
|
|
|
// @internal
|
|
export function getFromSessionStorage(key: string): null | string;
|
|
|
|
// @public
|
|
export function getHashForBuffer(buffer: ArrayBuffer): string;
|
|
|
|
// @public
|
|
export function getHashForObject(obj: any): string;
|
|
|
|
// @public
|
|
export function getHashForString(string: string): string;
|
|
|
|
// @public
|
|
export function getIndexAbove(below?: IndexKey | undefined): IndexKey;
|
|
|
|
// @public
|
|
export function getIndexBelow(above?: IndexKey | undefined): IndexKey;
|
|
|
|
// @public
|
|
export function getIndexBetween(below: IndexKey | undefined, above: IndexKey | undefined): IndexKey;
|
|
|
|
// @public
|
|
export function getIndices(n: number, start?: IndexKey): IndexKey[];
|
|
|
|
// @public
|
|
export function getIndicesAbove(below: IndexKey | undefined, n: number): IndexKey[];
|
|
|
|
// @public
|
|
export function getIndicesBelow(above: IndexKey | undefined, n: number): IndexKey[];
|
|
|
|
// @public
|
|
export function getIndicesBetween(below: IndexKey | undefined, above: IndexKey | undefined, n: number): IndexKey[];
|
|
|
|
// @internal (undocumented)
|
|
export function getOwnProperty<K extends string, V>(obj: Partial<Record<K, V>>, key: K): undefined | V;
|
|
|
|
// @internal (undocumented)
|
|
export function getOwnProperty(obj: object, key: string): unknown;
|
|
|
|
// @internal (undocumented)
|
|
export function hasOwnProperty(obj: object, key: string): boolean;
|
|
|
|
// @public
|
|
export type IndexKey = string & {
|
|
__orderKey: true;
|
|
};
|
|
|
|
// @public
|
|
export function invLerp(a: number, b: number, t: number): number;
|
|
|
|
// @public
|
|
export function isDefined<T>(value: T): value is typeof value extends undefined ? never : T;
|
|
|
|
// @internal (undocumented)
|
|
export const isNativeStructuredClone: boolean;
|
|
|
|
// @public
|
|
export function isNonNull<T>(value: T): value is typeof value extends null ? never : T;
|
|
|
|
// @public
|
|
export function isNonNullish<T>(value: T): value is typeof value extends undefined ? never : typeof value extends null ? never : T;
|
|
|
|
// @public (undocumented)
|
|
export type JsonArray = JsonValue[];
|
|
|
|
// @public (undocumented)
|
|
export interface JsonObject {
|
|
// (undocumented)
|
|
[key: string]: JsonValue | undefined;
|
|
}
|
|
|
|
// @public (undocumented)
|
|
export type JsonPrimitive = boolean | null | number | string;
|
|
|
|
// @public (undocumented)
|
|
export type JsonValue = JsonArray | JsonObject | JsonPrimitive;
|
|
|
|
// @internal (undocumented)
|
|
export function last<T>(arr: readonly T[]): T | undefined;
|
|
|
|
// @public
|
|
export function lerp(a: number, b: number, t: number): number;
|
|
|
|
// @public (undocumented)
|
|
export function lns(str: string): string;
|
|
|
|
// @internal
|
|
export function mapObjectMapValues<Key extends string, ValueBefore, ValueAfter>(object: {
|
|
readonly [K in Key]: ValueBefore;
|
|
}, mapper: (key: Key, value: ValueBefore) => ValueAfter): {
|
|
[K in Key]: ValueAfter;
|
|
};
|
|
|
|
// @internal (undocumented)
|
|
export function measureAverageDuration(_target: any, propertyKey: string, descriptor: PropertyDescriptor): PropertyDescriptor;
|
|
|
|
// @internal (undocumented)
|
|
export function measureCbDuration(name: string, cb: () => any): any;
|
|
|
|
// @internal (undocumented)
|
|
export function measureDuration(_target: any, propertyKey: string, descriptor: PropertyDescriptor): PropertyDescriptor;
|
|
|
|
// @public
|
|
export class MediaHelpers {
|
|
static getImageSize(blob: Blob): Promise<{
|
|
h: number;
|
|
w: number;
|
|
}>;
|
|
static getVideoSize(blob: Blob): Promise<{
|
|
h: number;
|
|
w: number;
|
|
}>;
|
|
// (undocumented)
|
|
static isAnimated(file: Blob): Promise<boolean>;
|
|
// (undocumented)
|
|
static isAnimatedImageType(mimeType: null | string): boolean;
|
|
// (undocumented)
|
|
static isImageType(mimeType: string): boolean;
|
|
// (undocumented)
|
|
static isStaticImageType(mimeType: null | string): boolean;
|
|
static loadImage(src: string): Promise<HTMLImageElement>;
|
|
static loadVideo(src: string): Promise<HTMLVideoElement>;
|
|
// (undocumented)
|
|
static usingObjectURL<T>(blob: Blob, fn: (url: string) => Promise<T>): Promise<T>;
|
|
}
|
|
|
|
// @internal (undocumented)
|
|
export function minBy<T>(arr: readonly T[], fn: (item: T) => number): T | undefined;
|
|
|
|
// @public
|
|
export function modulate(value: number, rangeA: number[], rangeB: number[], clamp?: boolean): number;
|
|
|
|
// @internal
|
|
export function noop(): void;
|
|
|
|
// @internal
|
|
export function objectMapEntries<Key extends string, Value>(object: {
|
|
[K in Key]: Value;
|
|
}): Array<[Key, Value]>;
|
|
|
|
// @internal
|
|
export function objectMapFromEntries<Key extends string, Value>(entries: ReadonlyArray<readonly [Key, Value]>): {
|
|
[K in Key]: Value;
|
|
};
|
|
|
|
// @internal
|
|
export function objectMapKeys<Key extends string>(object: {
|
|
readonly [K in Key]: unknown;
|
|
}): Array<Key>;
|
|
|
|
// @internal
|
|
export function objectMapValues<Key extends string, Value>(object: {
|
|
[K in Key]: Value;
|
|
}): Array<Value>;
|
|
|
|
// @public (undocumented)
|
|
export interface OkResult<T> {
|
|
// (undocumented)
|
|
readonly ok: true;
|
|
// (undocumented)
|
|
readonly value: T;
|
|
}
|
|
|
|
// @internal
|
|
export function omitFromStackTrace<Args extends Array<unknown>, Return>(fn: (...args: Args) => Return): (...args: Args) => Return;
|
|
|
|
// @internal
|
|
export function partition<T>(arr: T[], predicate: (item: T) => boolean): [T[], T[]];
|
|
|
|
// @public (undocumented)
|
|
export class PerformanceTracker {
|
|
// (undocumented)
|
|
isStarted(): boolean;
|
|
// (undocumented)
|
|
recordFrame: () => void;
|
|
// (undocumented)
|
|
start(name: string): void;
|
|
// (undocumented)
|
|
stop(): void;
|
|
}
|
|
|
|
// @public (undocumented)
|
|
export class PngHelpers {
|
|
// (undocumented)
|
|
static findChunk(view: DataView, type: string): {
|
|
dataOffset: number;
|
|
size: number;
|
|
start: number;
|
|
};
|
|
// (undocumented)
|
|
static getChunkType(view: DataView, offset: number): string;
|
|
// (undocumented)
|
|
static isPng(view: DataView, offset: number): boolean;
|
|
// (undocumented)
|
|
static parsePhys(view: DataView, offset: number): {
|
|
ppux: number;
|
|
ppuy: number;
|
|
unit: number;
|
|
};
|
|
// (undocumented)
|
|
static readChunks(view: DataView, offset?: number): Record<string, {
|
|
dataOffset: number;
|
|
size: number;
|
|
start: number;
|
|
}>;
|
|
// (undocumented)
|
|
static setPhysChunk(view: DataView, dpr?: number, options?: BlobPropertyBag): Blob;
|
|
}
|
|
|
|
// @internal (undocumented)
|
|
export function promiseWithResolve<T>(): Promise<T> & {
|
|
reject: (reason?: any) => void;
|
|
resolve: (value: T) => void;
|
|
};
|
|
|
|
// @public (undocumented)
|
|
export type RecursivePartial<T> = {
|
|
[P in keyof T]?: RecursivePartial<T[P]>;
|
|
};
|
|
|
|
// @internal (undocumented)
|
|
type Required_2<T, K extends keyof T> = Expand<Omit<T, K> & _Required<Pick<T, K>>>;
|
|
export { Required_2 as Required }
|
|
|
|
// @public (undocumented)
|
|
export type Result<T, E> = ErrorResult<E> | OkResult<T>;
|
|
|
|
// @public (undocumented)
|
|
export const Result: {
|
|
err<E>(error: E): ErrorResult<E>;
|
|
ok<T>(value: T): OkResult<T>;
|
|
};
|
|
|
|
// @public
|
|
export function rng(seed?: string): () => number;
|
|
|
|
// @public
|
|
export function rotateArray<T>(arr: T[], offset: number): T[];
|
|
|
|
// @internal
|
|
export function setInLocalStorage(key: string, value: string): void;
|
|
|
|
// @internal
|
|
export function setInSessionStorage(key: string, value: string): void;
|
|
|
|
// @public (undocumented)
|
|
export function sortById<T extends {
|
|
id: any;
|
|
}>(a: T, b: T): -1 | 1;
|
|
|
|
// @public
|
|
export function sortByIndex<T extends {
|
|
index: IndexKey;
|
|
}>(a: T, b: T): -1 | 0 | 1;
|
|
|
|
// @internal
|
|
export const STRUCTURED_CLONE_OBJECT_PROTOTYPE: any;
|
|
|
|
// @public
|
|
const structuredClone_2: <T>(i: T) => T;
|
|
export { structuredClone_2 as structuredClone }
|
|
|
|
// @public
|
|
export function throttle<T extends (...args: any) => any>(func: T, limit: number): (...args: Parameters<T>) => ReturnType<T>;
|
|
|
|
// @internal
|
|
export function throttleToNextFrame(fn: () => void): () => void;
|
|
|
|
// @internal (undocumented)
|
|
export function validateIndexKey(key: string): asserts key is IndexKey;
|
|
|
|
// @internal (undocumented)
|
|
export function warnDeprecatedGetter(name: string): void;
|
|
|
|
// @public
|
|
export class WeakCache<K extends object, V> {
|
|
get<P extends K>(item: P, cb: (item: P) => V): NonNullable<V>;
|
|
items: WeakMap<K, V>;
|
|
}
|
|
|
|
// @public
|
|
export const ZERO_INDEX_KEY: IndexKey;
|
|
|
|
// (No @packageDocumentation comment for this package)
|
|
|
|
```
|