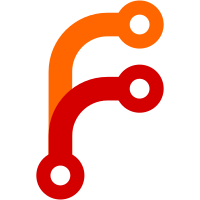
Based on #1549, but with a lot of code-structure related changes backed out. Shape schemas are still defined in tlschemas with this diff. Couple differences between this and #1549: - This tightens up the relationship between store schemas and editor schemas a bit - Reduces the number of places we need to remember to include core shapes - Only `<TLdrawEditor />` sets default shapes by default. If you're doing something funky with lower-level APIs, you need to specify `defaultShapes` manually - Replaces `validator` with `props` for shapes ### Change Type - [x] `major` — Breaking Change ### Test Plan 1. Add a step-by-step description of how to test your PR here. 2. - [x] Unit Tests - [ ] Webdriver tests ### Release Notes [dev-facing, notes to come]
204 lines
5.3 KiB
Markdown
204 lines
5.3 KiB
Markdown
## API Report File for "@tldraw/validate"
|
|
|
|
> Do not edit this file. It is a report generated by [API Extractor](https://api-extractor.com/).
|
|
|
|
```ts
|
|
|
|
// @public
|
|
const any: Validator<any>;
|
|
|
|
// @public
|
|
const array: Validator<unknown[]>;
|
|
|
|
// @public
|
|
function arrayOf<T>(itemValidator: Validatable<T>): ArrayOfValidator<T>;
|
|
|
|
// @public (undocumented)
|
|
class ArrayOfValidator<T> extends Validator<T[]> {
|
|
constructor(itemValidator: Validatable<T>);
|
|
// (undocumented)
|
|
readonly itemValidator: Validatable<T>;
|
|
// (undocumented)
|
|
lengthGreaterThan1(): Validator<T[]>;
|
|
// (undocumented)
|
|
nonEmpty(): Validator<T[]>;
|
|
}
|
|
|
|
// @public
|
|
const bigint: Validator<bigint>;
|
|
|
|
// @public
|
|
const boolean: Validator<boolean>;
|
|
|
|
// @public (undocumented)
|
|
const boxModel: ObjectValidator<{
|
|
x: number;
|
|
y: number;
|
|
w: number;
|
|
h: number;
|
|
}>;
|
|
|
|
// @public
|
|
function dict<Key extends string, Value>(keyValidator: Validatable<Key>, valueValidator: Validatable<Value>): DictValidator<Key, Value>;
|
|
|
|
// @public (undocumented)
|
|
class DictValidator<Key extends string, Value> extends Validator<Record<Key, Value>> {
|
|
constructor(keyValidator: Validatable<Key>, valueValidator: Validatable<Value>);
|
|
// (undocumented)
|
|
readonly keyValidator: Validatable<Key>;
|
|
// (undocumented)
|
|
readonly valueValidator: Validatable<Value>;
|
|
}
|
|
|
|
// @public
|
|
const integer: Validator<number>;
|
|
|
|
// @public
|
|
function literal<T extends boolean | number | string>(expectedValue: T): Validator<T>;
|
|
|
|
// @public
|
|
function model<T extends {
|
|
readonly id: string;
|
|
}>(name: string, validator: Validatable<T>): Validator<T>;
|
|
|
|
// @public
|
|
const nonZeroInteger: Validator<number>;
|
|
|
|
// @public
|
|
const nonZeroNumber: Validator<number>;
|
|
|
|
// @public
|
|
const number: Validator<number>;
|
|
|
|
// @public
|
|
function object<Shape extends object>(config: {
|
|
readonly [K in keyof Shape]: Validatable<Shape[K]>;
|
|
}): ObjectValidator<Shape>;
|
|
|
|
// @public (undocumented)
|
|
class ObjectValidator<Shape extends object> extends Validator<Shape> {
|
|
constructor(config: {
|
|
readonly [K in keyof Shape]: Validatable<Shape[K]>;
|
|
}, shouldAllowUnknownProperties?: boolean);
|
|
// (undocumented)
|
|
allowUnknownProperties(): ObjectValidator<Shape>;
|
|
// (undocumented)
|
|
readonly config: {
|
|
readonly [K in keyof Shape]: Validatable<Shape[K]>;
|
|
};
|
|
extend<Extension extends Record<string, unknown>>(extension: {
|
|
readonly [K in keyof Extension]: Validatable<Extension[K]>;
|
|
}): ObjectValidator<Shape & Extension>;
|
|
}
|
|
|
|
// @public (undocumented)
|
|
const point: ObjectValidator<{
|
|
x: number;
|
|
y: number;
|
|
z: number | undefined;
|
|
}>;
|
|
|
|
// @public
|
|
const positiveInteger: Validator<number>;
|
|
|
|
// @public
|
|
const positiveNumber: Validator<number>;
|
|
|
|
// @public (undocumented)
|
|
function setEnum<T>(values: ReadonlySet<T>): Validator<T>;
|
|
|
|
// @public
|
|
const string: Validator<string>;
|
|
|
|
declare namespace T {
|
|
export {
|
|
literal,
|
|
arrayOf,
|
|
object,
|
|
dict,
|
|
union,
|
|
model,
|
|
setEnum,
|
|
ValidatorFn,
|
|
Validatable,
|
|
ValidationError,
|
|
TypeOf,
|
|
Validator,
|
|
ArrayOfValidator,
|
|
ObjectValidator,
|
|
UnionValidator,
|
|
DictValidator,
|
|
unknown,
|
|
any,
|
|
string,
|
|
number,
|
|
positiveNumber,
|
|
nonZeroNumber,
|
|
integer,
|
|
positiveInteger,
|
|
nonZeroInteger,
|
|
boolean,
|
|
bigint,
|
|
array,
|
|
unknownObject,
|
|
point,
|
|
boxModel
|
|
}
|
|
}
|
|
export { T }
|
|
|
|
// @public (undocumented)
|
|
type TypeOf<V extends Validatable<unknown>> = V extends Validatable<infer T> ? T : never;
|
|
|
|
// @public
|
|
function union<Key extends string, Config extends UnionValidatorConfig<Key, Config>>(key: Key, config: Config): UnionValidator<Key, Config>;
|
|
|
|
// @public (undocumented)
|
|
class UnionValidator<Key extends string, Config extends UnionValidatorConfig<Key, Config>, UnknownValue = never> extends Validator<TypeOf<Config[keyof Config]> | UnknownValue> {
|
|
constructor(key: Key, config: Config, unknownValueValidation: (value: object, variant: string) => UnknownValue);
|
|
// (undocumented)
|
|
validateUnknownVariants<Unknown>(unknownValueValidation: (value: object, variant: string) => Unknown): UnionValidator<Key, Config, Unknown>;
|
|
}
|
|
|
|
// @public
|
|
const unknown: Validator<unknown>;
|
|
|
|
// @public (undocumented)
|
|
const unknownObject: Validator<Record<string, unknown>>;
|
|
|
|
// @public (undocumented)
|
|
type Validatable<T> = {
|
|
validate: (value: unknown) => T;
|
|
};
|
|
|
|
// @public (undocumented)
|
|
class ValidationError extends Error {
|
|
constructor(rawMessage: string, path?: ReadonlyArray<number | string>);
|
|
// (undocumented)
|
|
name: string;
|
|
// (undocumented)
|
|
readonly path: ReadonlyArray<number | string>;
|
|
// (undocumented)
|
|
readonly rawMessage: string;
|
|
}
|
|
|
|
// @public (undocumented)
|
|
class Validator<T> implements Validatable<T> {
|
|
constructor(validationFn: ValidatorFn<T>);
|
|
check(name: string, checkFn: (value: T) => void): Validator<T>;
|
|
// (undocumented)
|
|
check(checkFn: (value: T) => void): Validator<T>;
|
|
nullable(): Validator<null | T>;
|
|
optional(): Validator<T | undefined>;
|
|
refine<U>(otherValidationFn: (value: T) => U): Validator<U>;
|
|
validate(value: unknown): T;
|
|
// (undocumented)
|
|
readonly validationFn: ValidatorFn<T>;
|
|
}
|
|
|
|
// @public (undocumented)
|
|
type ValidatorFn<T> = (value: unknown) => T;
|
|
|
|
// (No @packageDocumentation comment for this package)
|
|
|
|
```
|