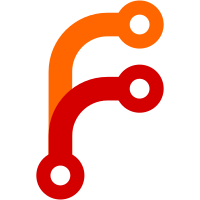
Switches from unpkg to our own cdn files. ### Change Type <!-- ❗ Please select a 'Scope' label ❗️ --> - [x] `sdk` — Changes the tldraw SDK - [ ] `dotcom` — Changes the tldraw.com web app - [ ] `docs` — Changes to the documentation, examples, or templates. - [ ] `vs code` — Changes to the vscode plugin - [ ] `internal` — Does not affect user-facing stuff <!-- ❗ Please select a 'Type' label ❗️ --> - [ ] `bugfix` — Bug fix - [ ] `feature` — New feature - [x] `improvement` — Improving existing features - [ ] `chore` — Updating dependencies, other boring stuff - [ ] `galaxy brain` — Architectural changes - [ ] `tests` — Changes to any test code - [ ] `tools` — Changes to infrastructure, CI, internal scripts, debugging tools, etc. - [ ] `dunno` — I don't know ### Release Notes - Start using our own cdn instead of unpkg.
72 lines
1.6 KiB
JavaScript
72 lines
1.6 KiB
JavaScript
require('fake-indexeddb/auto')
|
|
require('jest-canvas-mock')
|
|
global.ResizeObserver = require('resize-observer-polyfill')
|
|
global.crypto ??= new (require('@peculiar/webcrypto').Crypto)()
|
|
global.FontFace = class FontFace {
|
|
load() {
|
|
return Promise.resolve()
|
|
}
|
|
}
|
|
|
|
document.fonts = {
|
|
add: () => {},
|
|
delete: () => {},
|
|
forEach: () => {},
|
|
[Symbol.iterator]: () => [][Symbol.iterator](),
|
|
}
|
|
|
|
Object.defineProperty(window, 'matchMedia', {
|
|
writable: true,
|
|
value: jest.fn().mockImplementation((query) => ({
|
|
matches: false,
|
|
media: query,
|
|
onchange: null,
|
|
addListener: jest.fn(), // Deprecated
|
|
removeListener: jest.fn(), // Deprecated
|
|
addEventListener: jest.fn(),
|
|
removeEventListener: jest.fn(),
|
|
dispatchEvent: jest.fn(),
|
|
})),
|
|
})
|
|
|
|
Object.defineProperty(global.URL, 'createObjectURL', {
|
|
writable: true,
|
|
value: jest.fn(),
|
|
})
|
|
|
|
// Extract verson from package.json
|
|
const { version } = require('./package.json')
|
|
|
|
window.fetch = async (input, init) => {
|
|
if (input === `https://cdn.tldraw.com/${version}/translations/en.json`) {
|
|
const json = await import('@tldraw/assets/translations/main.json')
|
|
return {
|
|
ok: true,
|
|
json: async () => json.default,
|
|
}
|
|
}
|
|
|
|
if (input === '/icons/icon/icon-names.json') {
|
|
return {
|
|
ok: true,
|
|
json: async () => [],
|
|
}
|
|
}
|
|
|
|
throw new Error(`Unhandled request: ${input}`)
|
|
}
|
|
|
|
window.DOMRect = class DOMRect {
|
|
static fromRect(rect) {
|
|
return new DOMRect(rect.x, rect.y, rect.width, rect.height)
|
|
}
|
|
constructor(x, y, width, height) {
|
|
this.x = x
|
|
this.y = y
|
|
this.width = width
|
|
this.height = height
|
|
}
|
|
}
|
|
|
|
global.TextEncoder = require('util').TextEncoder
|
|
global.TextDecoder = require('util').TextDecoder
|