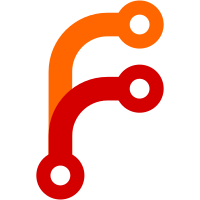
Typescript's type aliases (`type X = thing`) can refer to basically anything, which makes it hard to write an automatic document formatter for them. Interfaces on the other hand are only object, so they play much nicer with docs. Currently, object-flavoured type aliases don't really get expanded at all on our docs site, which means we have a bunch of docs content that's not shown on the site. This diff introduces a lint rule that forces `interface X {foo: bar}`s instead of `type X = {foo: bar}` where possible, as it results in a much better documentation experience: Before: <img width="437" alt="Screenshot 2024-05-22 at 15 24 13" src="https://github.com/tldraw/tldraw/assets/1489520/32606fd1-6832-4a1e-aa5f-f0534d160c92"> After: <img width="431" alt="Screenshot 2024-05-22 at 15 33 01" src="https://github.com/tldraw/tldraw/assets/1489520/4e0d59ee-c38e-4056-b9fd-6a7f15d28f0f"> ### Change Type - [x] `sdk` — Changes the tldraw SDK - [x] `docs` — Changes to the documentation, examples, or templates. - [x] `improvement` — Improving existing features
98 lines
2.7 KiB
TypeScript
98 lines
2.7 KiB
TypeScript
import { join } from 'path'
|
|
import { REPO_ROOT, writeJsonFile } from './file'
|
|
|
|
interface Label {
|
|
// this is what the label is 'called' on github
|
|
name: string
|
|
// this is how we describe the label in our pull request template
|
|
description: string
|
|
// this is the section title for the label in our changelogs
|
|
changelogTitle: string
|
|
}
|
|
|
|
const SCOPE_LABELS = [
|
|
{
|
|
name: `sdk`,
|
|
description: `Changes the tldraw SDK`,
|
|
changelogTitle: '📚 SDK Changes',
|
|
},
|
|
{
|
|
name: `dotcom`,
|
|
description: `Changes the tldraw.com web app`,
|
|
changelogTitle: '🖥️ tldraw.com Changes',
|
|
},
|
|
{
|
|
name: `docs`,
|
|
description: `Changes to the documentation, examples, or templates.`,
|
|
changelogTitle: '📖 Documentation changes',
|
|
},
|
|
{
|
|
name: `vs code`,
|
|
description: `Changes to the vscode plugin`,
|
|
changelogTitle: '👩💻 VS Code Plugin Changes',
|
|
},
|
|
{
|
|
name: `internal`,
|
|
description: `Does not affect user-facing stuff`,
|
|
changelogTitle: '🕵️♀️ Internal Changes',
|
|
},
|
|
] as const satisfies Label[]
|
|
|
|
const TYPE_LABELS = [
|
|
{ name: `bugfix`, description: `Bug fix`, changelogTitle: '🐛 Bug Fixes' },
|
|
{ name: `feature`, description: `New feature`, changelogTitle: '🚀 Features' },
|
|
{
|
|
name: `improvement`,
|
|
description: `Improving existing features`,
|
|
changelogTitle: '💄 Improvements',
|
|
},
|
|
{
|
|
name: `chore`,
|
|
description: `Updating dependencies, other boring stuff`,
|
|
changelogTitle: '🧹 Chores',
|
|
},
|
|
{
|
|
name: `galaxy brain`,
|
|
description: `Architectural changes`,
|
|
changelogTitle: '🤯 Architectural changes',
|
|
},
|
|
{ name: `tests`, description: `Changes to any test code`, changelogTitle: '🧪 Tests' },
|
|
{
|
|
name: `tools`,
|
|
description: `Changes to infrastructure, CI, internal scripts, debugging tools, etc.`,
|
|
changelogTitle: '🛠️ Tools',
|
|
},
|
|
{ name: `dunno`, description: `I don't know`, changelogTitle: '🤷 Dunno' },
|
|
] as const satisfies Label[]
|
|
|
|
export function getLabelNames() {
|
|
return [...SCOPE_LABELS, ...TYPE_LABELS].map((label) => label.name)
|
|
}
|
|
|
|
function formatTemplateOption(label: Label) {
|
|
return `- [ ] \`${label.name}\` — ${label.description}`
|
|
}
|
|
|
|
export function formatLabelOptionsForPRTemplate() {
|
|
let result = `<!-- ❗ Please select a 'Scope' label ❗️ -->\n\n`
|
|
for (const label of SCOPE_LABELS) {
|
|
result += formatTemplateOption(label) + '\n'
|
|
}
|
|
result += `\n<!-- ❗ Please select a 'Type' label ❗️ -->\n\n`
|
|
for (const label of TYPE_LABELS) {
|
|
result += formatTemplateOption(label) + '\n'
|
|
}
|
|
return result
|
|
}
|
|
|
|
export async function generateAutoRcFile() {
|
|
const autoRcPath = join(REPO_ROOT, '.autorc')
|
|
await writeJsonFile(autoRcPath, {
|
|
plugins: ['npm'],
|
|
labels: [...SCOPE_LABELS, ...TYPE_LABELS].map(({ name, changelogTitle }) => ({
|
|
name,
|
|
changelogTitle,
|
|
releaseType: 'none',
|
|
})),
|
|
})
|
|
}
|