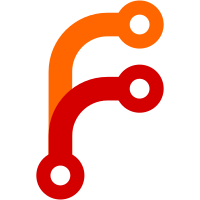
This PR starts putting in place the high-level changes we want to make to the docs site. - It makes separate sections for Reference and Examples and Community. - Gets rid of the secondary sidebar and integrates it into the main sidebar. - Groups the reference articles by type. - Pulls in the examples alongside code and a live playground so people don't have to visit examples.tldraw.com separately. <img width="1458" alt="Screenshot 2024-01-30 at 09 43 46" src="https://github.com/tldraw/tldraw/assets/469604/4f5aa339-3a69-4d9b-9b9f-dfdddea623e8"> Again, this is the top-level changes and there's more to be done for the next PR(s): - create quick start page - clean up installation page - add accordion to Examples page prbly - put fun stuff in header (from footer) - landing page - something for landing page of API - search cmd-k and border - cleanup _sidebarReferenceContentLinks - external links _blank - address potential skew issue with code examples - have a link to other examples (next.js, etc.) ### Change Type - [x] `documentation` — Changes to the documentation only[^2] ### Test Plan 1. Make sure examples work! ### Release Notes - Rework our docs site to pull together the examples app and reference section more cohesively. --------- Co-authored-by: Taha <98838967+Taha-Hassan-Git@users.noreply.github.com> Co-authored-by: Steve Ruiz <steveruizok@gmail.com> Co-authored-by: Mitja Bezenšek <mitja.bezensek@gmail.com> Co-authored-by: alex <alex@dytry.ch> Co-authored-by: Lu Wilson <l2wilson94@gmail.com> Co-authored-by: Dan Groshev <git@dgroshev.com>
93 lines
2.3 KiB
TypeScript
93 lines
2.3 KiB
TypeScript
import path from 'path'
|
|
import { open } from 'sqlite'
|
|
import sqlite3 from 'sqlite3'
|
|
|
|
export async function connect(opts = {} as { reset?: boolean }) {
|
|
const db = await open({
|
|
filename: path.join(process.cwd(), 'content.db'),
|
|
driver: sqlite3.Database,
|
|
})
|
|
|
|
if (opts.reset) {
|
|
// Create the authors table if it does not exist
|
|
|
|
await db.run(`DROP TABLE IF EXISTS authors`)
|
|
await db.run(`CREATE TABLE IF NOT EXISTS authors (
|
|
id TEXT PRIMARY_KEY,
|
|
name TEXT NOT NULL,
|
|
email TEXT NOT NULL,
|
|
twitter TEXT NOT NULL,
|
|
image TEXT NOT NULL
|
|
)`)
|
|
|
|
// Create the sections table if it does not exist
|
|
|
|
await db.run(`DROP TABLE IF EXISTS sections`)
|
|
await db.run(`CREATE TABLE sections (
|
|
id TEXT PRIMARY KEY,
|
|
idx INTEGER NOT NULL,
|
|
title TEXT NOT NULL,
|
|
path TEXT NOT NULL,
|
|
description TEXT,
|
|
sidebar_behavior TEXT
|
|
)`)
|
|
|
|
// Create the categories table if it does not exist
|
|
|
|
await db.run(`DROP TABLE IF EXISTS categories`)
|
|
await db.run(`CREATE TABLE IF NOT EXISTS categories (
|
|
id TEXT PRIMARY KEY,
|
|
title TEXT NOT NULL,
|
|
description TEXT,
|
|
sectionId TEXT NOT NULL,
|
|
sectionIndex INTEGER NOT NULL,
|
|
path TEXT,
|
|
FOREIGN KEY (id) REFERENCES sections(id)
|
|
)`)
|
|
|
|
// Create the articles table if it does not exist
|
|
|
|
// drop the table if it exists
|
|
|
|
await db.run(`DROP TABLE IF EXISTS articles`)
|
|
await db.run(`CREATE TABLE IF NOT EXISTS articles (
|
|
id TEXT PRIMARY KEY,
|
|
groupIndex INTEGER NOT NULL,
|
|
categoryIndex INTEGER NOT NULL,
|
|
sectionIndex INTEGER NOT NULL,
|
|
groupId TEXT,
|
|
categoryId TEXT NOT NULL,
|
|
sectionId TEXT NOT NULL,
|
|
authorId TEXT NOT NULL,
|
|
title TEXT NOT NULL,
|
|
description TEXT,
|
|
hero TEXT,
|
|
status TEXT NOT NULL,
|
|
date TEXT,
|
|
sourceUrl TEXT,
|
|
componentCode TEXT,
|
|
componentCodeFiles TEXT,
|
|
keywords TEXT,
|
|
content TEXT NOT NULL,
|
|
path TEXT,
|
|
FOREIGN KEY (authorId) REFERENCES authors(id),
|
|
FOREIGN KEY (sectionId) REFERENCES sections(id),
|
|
FOREIGN KEY (categoryId) REFERENCES categories(id)
|
|
)`)
|
|
|
|
await db.run(`DROP TABLE IF EXISTS headings`)
|
|
await db.run(`CREATE TABLE IF NOT EXISTS headings (
|
|
id INTEGER PRIMARY KEY AUTOINCREMENT,
|
|
idx INTEGER NOT NULL,
|
|
articleId TEXT NOT NULL,
|
|
level INTEGER NOT NULL,
|
|
title TEXT NOT NULL,
|
|
slug TEXT NOT NULL,
|
|
isCode BOOL NOT NULL,
|
|
path TEXT NOT NULL,
|
|
FOREIGN KEY (articleId) REFERENCES articles(id)
|
|
)`)
|
|
}
|
|
|
|
return db
|
|
}
|