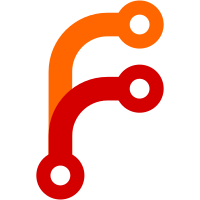
* Added exporting of shapeses * added video serialization * Fix viewport sizes, add chrome-aws-lambda for puppeteer * Update menu styling * extract to callback * Update Loading.tsx * force update menu * fix missing fonts * Added SVG and JSON export * Fix json exports * Merge branch 'main' into pr/468, update menus * Update TldrawApp.ts Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
55 lines
1.3 KiB
TypeScript
55 lines
1.3 KiB
TypeScript
import React from 'react'
|
|
import * as gtag from 'utils/gtag'
|
|
import { Tldraw, TldrawApp, TldrawProps, useFileSystem } from '@tldraw/tldraw'
|
|
import { useAccountHandlers } from 'hooks/useAccountHandlers'
|
|
import { exportToImage } from 'utils/export'
|
|
|
|
declare const window: Window & { app: TldrawApp }
|
|
|
|
interface EditorProps {
|
|
id?: string
|
|
isUser?: boolean
|
|
isSponsor?: boolean
|
|
}
|
|
|
|
export default function Editor({
|
|
id = 'home',
|
|
isUser = false,
|
|
isSponsor = false,
|
|
...rest
|
|
}: EditorProps & Partial<TldrawProps>) {
|
|
const handleMount = React.useCallback((app: TldrawApp) => {
|
|
window.app = app
|
|
}, [])
|
|
|
|
// Send events to gtag as actions.
|
|
const handlePersist = React.useCallback((_app: TldrawApp, reason?: string) => {
|
|
gtag.event({
|
|
action: reason ?? '',
|
|
category: 'editor',
|
|
label: reason ?? 'persist',
|
|
value: 0,
|
|
})
|
|
}, [])
|
|
|
|
const fileSystemEvents = useFileSystem()
|
|
|
|
const { onSignIn, onSignOut } = useAccountHandlers()
|
|
|
|
return (
|
|
<div className="tldraw">
|
|
<Tldraw
|
|
id={id}
|
|
autofocus
|
|
onMount={handleMount}
|
|
onPersist={handlePersist}
|
|
showSponsorLink={!isSponsor}
|
|
onSignIn={isSponsor ? undefined : onSignIn}
|
|
onSignOut={isUser ? onSignOut : undefined}
|
|
onExport={exportToImage}
|
|
{...fileSystemEvents}
|
|
{...rest}
|
|
/>
|
|
</div>
|
|
)
|
|
}
|