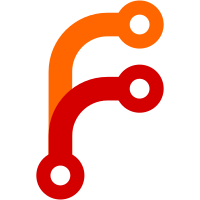
This PR aims to make sure #4030 doesn't need to happen again. We check that the worker file sizes stay within a given limit, and require people to explicitly up this limit if they decide to add new deps that grow the bundle size significantly. ### Change Type <!-- ❗ Please select a 'Type' label ❗️ --> - [ ] `feature` — New feature - [ ] `improvement` — Product improvement - [ ] `api` — API change - [ ] `bugfix` — Bug fix - [x] `other` — Changes that don't affect SDK users, e.g. internal or .com changes ### Test Plan 1. Add a step-by-step description of how to test your PR here. 2. - [ ] Unit Tests - [ ] End to end tests ### Release Notes - Add a brief release note for your PR here.
63 lines
1.6 KiB
TypeScript
63 lines
1.6 KiB
TypeScript
import { existsSync, readFileSync, rmSync } from 'fs'
|
|
import kleur from 'kleur'
|
|
import minimist from 'minimist'
|
|
import { resolve } from 'path'
|
|
import { exec } from './lib/exec'
|
|
|
|
const args = minimist(process.argv.slice(2))
|
|
const sizeLimit = Number(args['size-limit-bytes'])
|
|
if (!isFinite(sizeLimit) || sizeLimit < 1) {
|
|
console.error(
|
|
'Invalid usage. Usage: yarn tsx check-worker-bundle.ts --size-limit-bytes <size> --entry <entry>'
|
|
)
|
|
process.exit(1)
|
|
}
|
|
|
|
const entry = args['entry']
|
|
if (!existsSync(entry)) {
|
|
console.error('Entry file does not exist:', entry)
|
|
process.exit(1)
|
|
}
|
|
|
|
const bundleMetaFileName = '.bundle-meta.json'
|
|
|
|
interface Meta {
|
|
outputs: {
|
|
[fileName: string]: {
|
|
bytes: number
|
|
}
|
|
}
|
|
}
|
|
|
|
async function checkBundleSize() {
|
|
rmSync(bundleMetaFileName, { force: true })
|
|
|
|
await exec('yarn', [
|
|
'esbuild',
|
|
entry,
|
|
'--bundle',
|
|
'--outfile=/dev/null',
|
|
'--minify',
|
|
'--external:cloudflare:workers',
|
|
'--metafile=' + bundleMetaFileName,
|
|
])
|
|
|
|
console.log(kleur.cyan().bold('Checking bundle size'), 'of', resolve(entry) + '\n')
|
|
|
|
const meta = JSON.parse(readFileSync(bundleMetaFileName).toString()) as Meta
|
|
const totalSize = Object.values(meta.outputs).reduce((acc, output) => acc + output.bytes, 0)
|
|
if (totalSize === 0) {
|
|
throw new Error('Invalid bundle meta in ' + process.cwd() + '/' + bundleMetaFileName)
|
|
}
|
|
|
|
if (totalSize > sizeLimit) {
|
|
console.error(
|
|
`${kleur.red().bold('ERROR')} Bundle size exceeds limit: ${totalSize} > ${sizeLimit}`
|
|
)
|
|
process.exit(1)
|
|
}
|
|
|
|
console.log(`Bundle size is within limit: ${totalSize} < ${sizeLimit}`)
|
|
}
|
|
|
|
checkBundleSize()
|