This PR is a significant rewrite of our selection / hit testing logic.
It
- replaces our current geometric helpers (`getBounds`, `getOutline`,
`hitTestPoint`, and `hitTestLineSegment`) with a new geometry API
- moves our hit testing entirely to JS using geometry
- improves selection logic, especially around editing shapes, groups and
frames
- fixes many minor selection bugs (e.g. shapes behind frames)
- removes hit-testing DOM elements from ShapeFill etc.
- adds many new tests around selection
- adds new tests around selection
- makes several superficial changes to surface editor APIs
This PR is hard to evaluate. The `selection-omnibus` test suite is
intended to describe all of the selection behavior, however all existing
tests are also either here preserved and passing or (in a few cases
around editing shapes) are modified to reflect the new behavior.
## Geometry
All `ShapeUtils` implement `getGeometry`, which returns a single
geometry primitive (`Geometry2d`). For example:
```ts
class BoxyShapeUtil {
getGeometry(shape: BoxyShape) {
return new Rectangle2d({
width: shape.props.width,
height: shape.props.height,
isFilled: true,
margin: shape.props.strokeWidth
})
}
}
```
This geometric primitive is used for all bounds calculation, hit
testing, intersection with arrows, etc.
There are several geometric primitives that extend `Geometry2d`:
- `Arc2d`
- `Circle2d`
- `CubicBezier2d`
- `CubicSpline2d`
- `Edge2d`
- `Ellipse2d`
- `Group2d`
- `Polygon2d`
- `Rectangle2d`
- `Stadium2d`
For shapes that have more complicated geometric representations, such as
an arrow with a label, the `Group2d` can accept other primitives as its
children.
## Hit testing
Previously, we did all hit testing via events set on shapes and other
elements. In this PR, I've replaced those hit tests with our own
calculation for hit tests in JavaScript. This removed the need for many
DOM elements, such as hit test area borders and fills which only existed
to trigger pointer events.
## Selection
We now support selecting "hollow" shapes by clicking inside of them.
This involves a lot of new logic but it should work intuitively. See
`Editor.getShapeAtPoint` for the (thoroughly commented) implementation.

every sunset is actually the sun hiding in fear and respect of tldraw's
quality of interactions
This PR also fixes several bugs with scribble selection, in particular
around the shift key modifier.
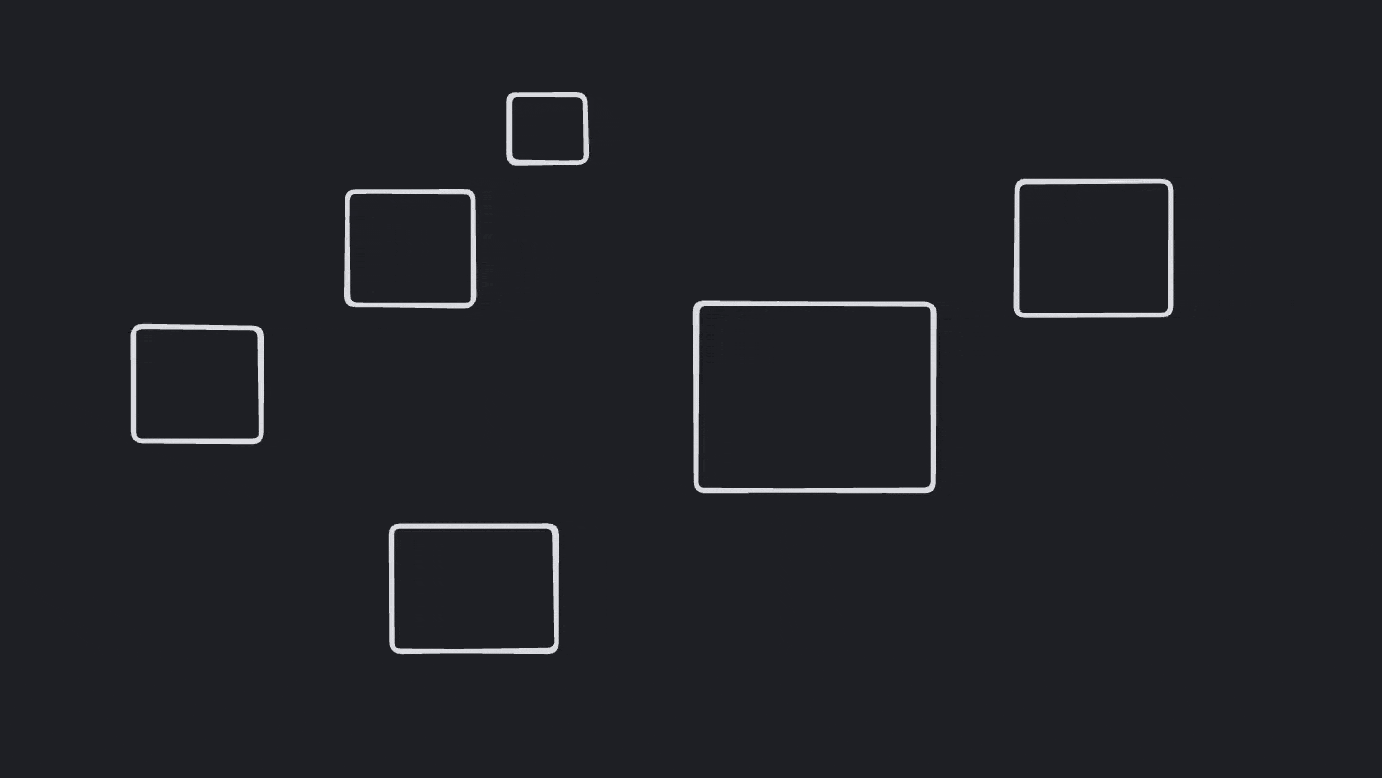
...as well as issues with labels and editing.
There are **over 100 new tests** for selection covering groups, frames,
brushing, scribbling, hovering, and editing. I'll add a few more before
I feel comfortable merging this PR.
## Arrow binding
Using the same "hollow shape" logic as selection, arrow binding is
significantly improved.

a thousand wise men could not improve on this
## Moving focus between editing shapes
Previously, this was handled in the `editing_shapes` state. This is
moved to `useEditableText`, and should generally be considered an
advanced implementation detail on a shape-by-shape basis. This addresses
a bug that I'd never noticed before, but which can be reproduced by
selecting an shape—but not focusing its input—while editing a different
shape. Previously, the new shape became the editing shape but its input
did not focus.
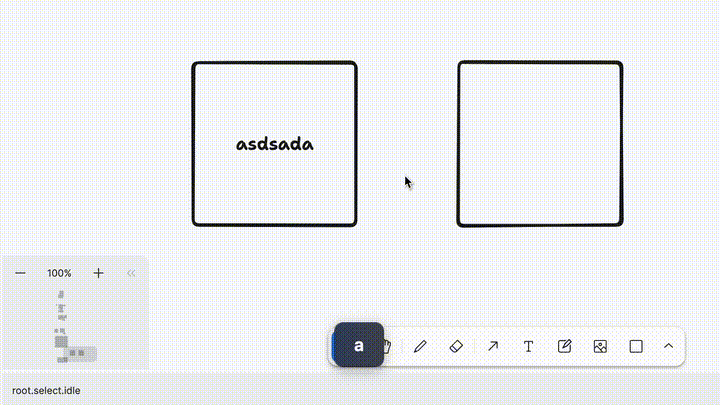
In this PR, you can select a shape by clicking on its edge or body, or
select its input to transfer editing / focus.

tldraw, glorious tldraw
### Change Type
- [x] `major` — Breaking change
### Test Plan
1. Erase shapes
2. Select shapes
3. Calculate their bounding boxes
- [ ] Unit Tests // todo
- [ ] End to end tests // todo
### Release Notes
- [editor] Remove `ShapeUtil.getBounds`, `ShapeUtil.getOutline`,
`ShapeUtil.hitTestPoint`, `ShapeUtil.hitTestLineSegment`
- [editor] Add `ShapeUtil.getGeometry`
- [editor] Add `Editor.getShapeGeometry`
This PR fixes snapping for arrow shapes. Previously, the middle handle
of an arrow was marked as a vertex, causing the arrow to have to
segments (one of which would be snapped to). In this PR we make the
second handle a "virtual" handle and tweak how we display handles to
preserve the same appearance.
### Change Type
- [x] `minor` — New feature
### Test Plan
1. Drag an arrow while snapping.
### Release Notes
- [fix] arrow snapping
This PR is another grab bag:
- renames `readOnly` to `readonly` throughout editor
- fixes a regression related to focus and keyboard shortcuts
- adds a small outline for focused editors
### Change Type
- [x] `major`
### Test Plan
- [x] End to end tests
This PR moves code between our packages so that:
- @tldraw/editor is a “core” library with the engine and canvas but no
shapes, tools, or other things
- @tldraw/tldraw contains everything particular to the experience we’ve
built for tldraw
At first look, this might seem like a step away from customization and
configuration, however I believe it greatly increases the configuration
potential of the @tldraw/editor while also providing a more accurate
reflection of what configuration options actually exist for
@tldraw/tldraw.
## Library changes
@tldraw/editor re-exports its dependencies and @tldraw/tldraw re-exports
@tldraw/editor.
- users of @tldraw/editor WITHOUT @tldraw/tldraw should almost always
only import things from @tldraw/editor.
- users of @tldraw/tldraw should almost always only import things from
@tldraw/tldraw.
- @tldraw/polyfills is merged into @tldraw/editor
- @tldraw/indices is merged into @tldraw/editor
- @tldraw/primitives is merged mostly into @tldraw/editor, partially
into @tldraw/tldraw
- @tldraw/file-format is merged into @tldraw/tldraw
- @tldraw/ui is merged into @tldraw/tldraw
Many (many) utils and other code is moved from the editor to tldraw. For
example, embeds now are entirely an feature of @tldraw/tldraw. The only
big chunk of code left in core is related to arrow handling.
## API Changes
The editor can now be used without tldraw's assets. We load them in
@tldraw/tldraw instead, so feel free to use whatever fonts or images or
whatever that you like with the editor.
All tools and shapes (except for the `Group` shape) are moved to
@tldraw/tldraw. This includes the `select` tool.
You should use the editor with at least one tool, however, so you now
also need to send in an `initialState` prop to the Editor /
<TldrawEditor> component indicating which state the editor should begin
in.
The `components` prop now also accepts `SelectionForeground`.
The complex selection component that we use for tldraw is moved to
@tldraw/tldraw. The default component is quite basic but can easily be
replaced via the `components` prop. We pass down our tldraw-flavored
SelectionFg via `components`.
Likewise with the `Scribble` component: the `DefaultScribble` no longer
uses our freehand tech and is a simple path instead. We pass down the
tldraw-flavored scribble via `components`.
The `ExternalContentManager` (`Editor.externalContentManager`) is
removed and replaced with a mapping of types to handlers.
- Register new content handlers with
`Editor.registerExternalContentHandler`.
- Register new asset creation handlers (for files and URLs) with
`Editor.registerExternalAssetHandler`
### Change Type
- [x] `major` — Breaking change
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
- [@tldraw/editor] lots, wip
- [@tldraw/ui] gone, merged to tldraw/tldraw
- [@tldraw/polyfills] gone, merged to tldraw/editor
- [@tldraw/primitives] gone, merged to tldraw/editor / tldraw/tldraw
- [@tldraw/indices] gone, merged to tldraw/editor
- [@tldraw/file-format] gone, merged to tldraw/tldraw
---------
Co-authored-by: alex <alex@dytry.ch>
This PR adds `box-sizing: border-box` to the editor and its children.
### Change Type
- [x] `patch`
### Release Notes
- [@tldraw/editor] Add `box-sizing: border-box` to `tl-container`
tldraw-zero themed follow-ups to the styles API added in #1580.
- Removed style related helpers from `ShapeUtil`
- `editor.css` no longer includes the tldraw default color palette.
Instead, a global `DefaultColorPalette` is defined as part of the color
style. If developers wish to cusomise the colors, they can mutate that
global.
- `ShapeUtil.toSvg` no longer takes font/color. Instead, it takes an
"svg export context" that can be used to add `<defs>` to the exported
SVG element. Converting e.g. fonts to inlined data urls is now the
responsibility of the shapes that use them rather than the Editor.
- `usePattern` is not longer a core part of the editor. Instead,
`ShapeUtil` has a `getCanvasSvgDefs` method for returning react
components representing anything a shape needs included in `<defs>` for
the canvas.
- The shape-specific cleanup logic in `setStyle` has been deleted. It
turned out that none of that logic has been running anyway, and instead
the relevant logic lives in shape `onBeforeChange` callbacks already.
### Change Type
- [x] `minor` — New feature
### Test Plan
- [x] Unit Tests
- [x] End to end tests
### Release Notes
--
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
This PR fixes a bug where our custom SVG cursors were not being used,
causing cursor chat to look worse on Windows.
It was the result of a dodgy merge!
### Change Type
- [x] `patch` — Bug fix
[^1]: publishes a `patch` release, for devDependencies use `internal`
[^2]: will not publish a new version
### Test Plan
To test this, you might need to change your OS cursor to something
different to usual. I set mine to off-black so that I can test this sort
of thing.
1. Make sure that your cursor is our custom black cursor when using the
app.
- [ ] Unit Tests
- [ ] End to end tests
### Release Notes
- None: Fixing an unreleased bug.
This PR introduces `@tldraw/tldraw/tldraw.css`, an exported CSS file
that replaces the `editor.css` and `ui.css` that were previously copied
from the editor and ui packages. Instead, these files are combined into
the `tldraw.css` file, simplifying the import story when using
`@tldraw/tldraw`.
### Change Type
- [x] `major` — Breaking change
### Release Notes
- [tldraw] Removes `editor.css` and `ui.css` exports, replaces with
`tldraw.css`
This PR adds support for seeing **another user**'s chat messages.
It's part 1 of two PRs relating to Cursor Chat.
And it's needed for the much bigger part 2:
https://github.com/tldraw/brivate/pull/1981
# Presence
You can see another person's chat messages!
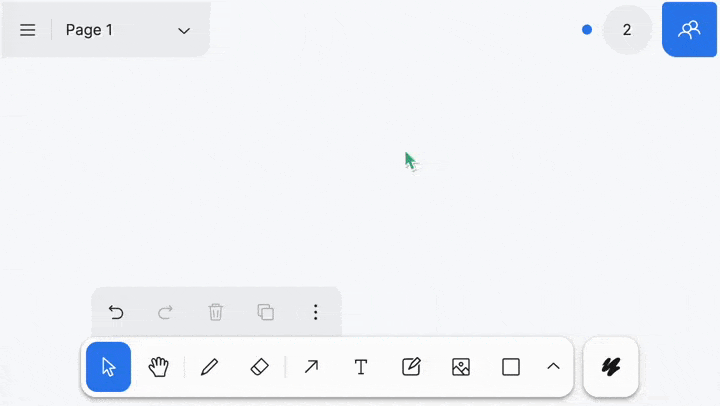
If they have a name, it gets popped on top.

That's it!
With this PR, there's no way of actually *typing* your chat messages.
That comes with the [next
one](https://github.com/tldraw/brivate/pull/1981)!
# Admin
### To-do
- [x] Store chat message
- [x] Allow overflowing chat
- [x] Presence for chat message
- [x] Display chat message to others
### Change Type
- [x] `minor` — New Feature
### Test Plan
To test this, I recommend checking out both `lu/cursor-chat` branches,
and opening two browser sessions in the same shared project.
1. In one session, type some cursor chat by pressing the Enter key while
on the canvas (and typing).
2. On the other session, check that you can see the chat message appear.
3. Repeat this while being both named, and unnamed.
I recommend just focusing on the visible presense in this PR.
The [other PR](https://github.com/tldraw/brivate/pull/1981) is where we
can focus about how we _input_ the cursor chat.
### Release Notes
- [dev] Added support for cursor chat presence.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
We load the user preferences a bit earlier, so that we can make sure
that the `LoadingScreen` and `ErrorScreen` also use the correct color
and background color based on the dark mode setting.
There's still a brief flash of white screen, but that's before any of
our components load, not sure if we can avoid that one.
Solves https://github.com/tldraw/tldraw/issues/1248
### Change Type
- [x] `patch` — Bug Fix
### Test Plan
1. Probably best if you throttle your network speed.
2. Reload the page.
3. The asset loading screen should use take your dark mode setting into
account.
4. Change the dark mode and try again.
### Release Notes
- Make sure our loading and error screens take dark mode setting into
account.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
This PR simplifies the static cursors.
### Change Type
- [x] `internal` — Any other changes that don't affect the published
package (will not publish a new version)
### Test Plan
1. Use cursors throughout app.
### Release Notes
- (editor) Simplifies the cursors in our CSS.
This PR adds a colored box around the current window when following
another user.
### Change Type
- [x] `minor` — New Feature
### Test Plan
1. Follow a user
2. Check out the box
### Release Notes
- Adds viewport following indicator
This adds a migration to migrate existing alignment options to their
legacy counter parts (`start` -> `start-legacy`, `end` -> `end-legacy`,
`middle` -> `middle-legacy`).
With this change the legacy options don't show any align as active in
the Styles panel:

I think this is probably what we want.
### Change Type
- [x] `patch` — Bug Fix
### Test Plan
1. Use some old preview link to create Geo and Note shapes with old
alignment options. You can use this one:
https://examples-kzwtf68jr-tldraw.vercel.app/
2. Copy and paste these shapes over to staging. Nothing should change
visually.
3. Also try out exporting to svg (with both old and new alignment
options)
- [x] Unit Tests
- [ ] Webdriver tests
### Release Notes
- Add support for legacy alignment options.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
Fixes#1410
This PR adds custom SVGs for all cursor types.
This will unblock some upcoming collaboration features!
It also adds some basic debugging for custom cursors.

It uses custom cursors for any shape-related UI, like links.

But it sticks with the default browser cursors for the non-canvas UI.

### Change Type
- [x] `minor`
### Test Plan
1. Enable debug mode.
2. From the debug menu, enable "Debug cursors".
3. Hover the cursor over the shapes that appear.
4. Check that the cursor appears correctly over each one.
5. (Don't forget to turn off "Debug cursors" after use).
### Release Notes
- Added consistent custom cursors.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
This PR makes it so that horizontal alignment in geo and sticky note
shapes also effects the position of the text within the shape.
<img width="1169" alt="image"
src="https://github.com/tldraw/tldraw/assets/23072548/96b28a7d-0f13-46ba-9ea1-82d02b4f870b">
<img width="1274" alt="image"
src="https://github.com/tldraw/tldraw/assets/23072548/fa768c71-4e9e-4cfe-ad8a-94d7700c445d">
This PR also places the shape's label at the center when there is no
text and the shape is not editing.
### Change Type
- [x] `minor` — New Feature
### Test Plan
1. Create shapes with labels
2. Confirm that their labels are positioned correctly
3. Export the shapes and verify the export
### Release Notes
- Geo shapes and sticky notes now position their labels based on their
alignment.
This PR adds a laser pointer. It's also available in readonly rooms.
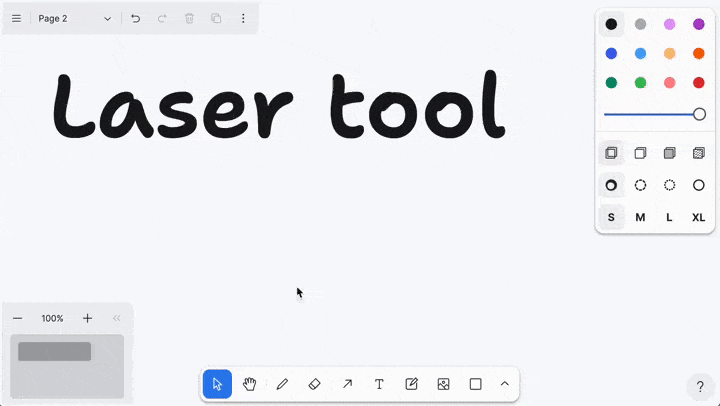
### Change Type
- [x] `minor` — New Feature
### Test Plan
1. Select the laser pointer tool
2. Draw some lasers.
### Release Notes
- Adds the laser pointer tool.
Vertical text alignment for geo shapes.
### Change Type
- [x] `minor` — New Feature
### Test Plan
1. Add a step-by-step description of how to test your PR here.
2.
- [ ] Unit Tests
- [ ] Webdriver tests
### Release Notes
- This adds vertical text alignment property to geo shapes.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
This PR fixes several issues with the way that SVG overlays were
rendered.
- fixes editing embed shape on firefox (weird SVG pointer events bug)
- fixes layering of overlays
- collaborator cursors are offset
### Change Type
- [x] `patch` — change to unshipped changes
### Test Plan
1. Try editing an embed shape on Firefox
2. Confirm that cursor hints are no longer spinning
3. Confirm that cursors are displayed correctly over other shapes
Nasty one!
This PR fixes the selection foreground of shapes getting misaligned when
the browser zoom was set to something other than 100%. It was always
happening all the time on android.

### Change Type
- [x] `patch` — Bug Fix
### Test Plan
1. Make a shape.
2. Select it.
3. Change your browser's zoom level.
4. Make sure the selection foreground stay in the right place (eg:
indicator, resize handles).
### Release Notes
- None (fix for a bug that hasn't released)
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>
This PR has been hijacked! 🗑️🦝🦝🦝
The <Canvas> component was previously split into an <SVGLayer> and an
<HTMLLayer>, mainly due to the complexity around translating SVGs.
However, this was done before we learned that SVGs can have overflow:
visible, so it turns out that we don't really need the SVGLayer at all.
This PR now refactors away SVG Layer.
It also updates the class name prefix in editor from `rs-` to `tl-` and
does a few other small changes.
---------
Co-authored-by: Steve Ruiz <steveruizok@gmail.com>