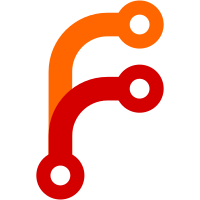
Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> Co-authored-by: Pranav Raj S <pranav@chatwoot.com>
28 lines
1 KiB
JavaScript
28 lines
1 KiB
JavaScript
import axios from 'axios';
|
|
import { actions } from '../../bulkActions';
|
|
import * as types from '../../../mutation-types';
|
|
import payload from './fixtures';
|
|
const commit = jest.fn();
|
|
global.axios = axios;
|
|
jest.mock('axios');
|
|
|
|
describe('#actions', () => {
|
|
describe('#create', () => {
|
|
it('sends correct actions if API is success', async () => {
|
|
axios.post.mockResolvedValue({ data: payload });
|
|
await actions.process({ commit }, payload);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_BULK_ACTIONS_FLAG, { isUpdating: true }],
|
|
[types.default.SET_BULK_ACTIONS_FLAG, { isUpdating: false }],
|
|
]);
|
|
});
|
|
it('sends correct actions if API is error', async () => {
|
|
axios.post.mockRejectedValue({ message: 'Incorrect header' });
|
|
await expect(actions.process({ commit })).rejects.toThrow(Error);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_BULK_ACTIONS_FLAG, { isUpdating: true }],
|
|
[types.default.SET_BULK_ACTIONS_FLAG, { isUpdating: false }],
|
|
]);
|
|
});
|
|
});
|
|
});
|