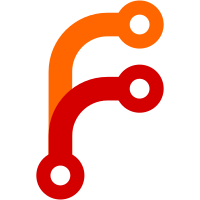
* Enhancement: Updates sidebar to a new design (#2733) * feat: Changes primary navbar to new design (#2598) * feat: updates design for secondary navbar (#2612) * Changes primary nvbar to new design * Updates design for contexual sidebar * Fixes issues with JSON * Remove duplication of notificatons in Navigation * Fixes broken tests * Fixes broken tests * Update app/javascript/dashboard/components/layout/AvailabilityStatus.vue * Update app/javascript/dashboard/components/layout/AvailabilityStatus.vue * Update app/javascript/dashboard/components/layout/SidebarItem.vue Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> * Update app/javascript/dashboard/components/layout/SidebarItem.vue Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> * Update app/javascript/dashboard/modules/sidebar/components/Secondary.vue Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> * Chore: Update design changes to features * Fixes menu transitions and refactors code * Refactors sidebar routeing logic * lint error fixes * Fixes dropdown menu styles * Fixes secondary new item links * Fixes lint scss issues * fixes linter issues * Fixes broken test cases * Update AvailabilityStatus.spec.js * Review feedbacks * Fixes add modal for label * Add tooltip for primary menu item * Tooltip for notifications * Adds tooltip for primary menu items * Review fixes * Review fixes * Fix merge issues * fixes logo size for login pages * fixes Merge breaks with styles Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com> Co-authored-by: Pranav Raj S <pranav@chatwoot.com>
70 lines
1.7 KiB
JavaScript
70 lines
1.7 KiB
JavaScript
import AgentDetails from '../AgentDetails';
|
|
import { createLocalVue, shallowMount } from '@vue/test-utils';
|
|
import Vuex from 'vuex';
|
|
import VueI18n from 'vue-i18n';
|
|
import VTooltip from 'v-tooltip';
|
|
|
|
import i18n from 'dashboard/i18n';
|
|
import Thumbnail from 'dashboard/components/widgets/Thumbnail';
|
|
import WootButton from 'dashboard/components/ui/WootButton';
|
|
const localVue = createLocalVue();
|
|
localVue.use(Vuex);
|
|
localVue.use(VueI18n);
|
|
localVue.component('thumbnail', Thumbnail);
|
|
localVue.component('woot-button', WootButton);
|
|
localVue.component('woot-button', WootButton);
|
|
localVue.use(VTooltip, {
|
|
defaultHtml: false,
|
|
});
|
|
|
|
const i18nConfig = new VueI18n({
|
|
locale: 'en',
|
|
messages: i18n,
|
|
});
|
|
|
|
describe('agentDetails', () => {
|
|
const currentUser = {
|
|
name: 'Neymar Junior',
|
|
avatar_url: '',
|
|
availability_status: 'online',
|
|
};
|
|
const currentRole = 'agent';
|
|
let store = null;
|
|
let actions = null;
|
|
let modules = null;
|
|
let agentDetails = null;
|
|
|
|
beforeEach(() => {
|
|
actions = {};
|
|
|
|
modules = {
|
|
auth: {
|
|
getters: {
|
|
getCurrentUser: () => currentUser,
|
|
getCurrentRole: () => currentRole,
|
|
getCurrentUserAvailability: () => currentUser.availability_status,
|
|
},
|
|
},
|
|
};
|
|
|
|
store = new Vuex.Store({
|
|
actions,
|
|
modules,
|
|
});
|
|
|
|
agentDetails = shallowMount(AgentDetails, {
|
|
store,
|
|
localVue,
|
|
i18n: i18nConfig,
|
|
});
|
|
});
|
|
|
|
it(' the agent status', () => {
|
|
expect(agentDetails.find('thumbnail-stub').vm.status).toBe('online');
|
|
});
|
|
|
|
it('agent thumbnail exists', () => {
|
|
const thumbnailComponent = agentDetails.findComponent(Thumbnail);
|
|
expect(thumbnailComponent.exists()).toBe(true);
|
|
});
|
|
});
|