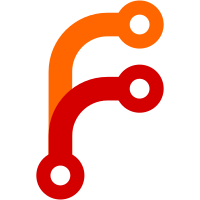
* feat: Search messages by content * Fix search UI * Add specs * chore: Filter search results * Update highlight logic * Rename query to searchTerm Co-authored-by: Sojan <sojan@pepalo.com>
44 lines
1.6 KiB
JavaScript
44 lines
1.6 KiB
JavaScript
import { actions } from '../../conversationSearch';
|
|
import types from '../../../mutation-types';
|
|
import axios from 'axios';
|
|
const commit = jest.fn();
|
|
global.axios = axios;
|
|
jest.mock('axios');
|
|
|
|
describe('#actions', () => {
|
|
describe('#get', () => {
|
|
it('sends correct actions if no query param is provided', () => {
|
|
actions.get({ commit }, { q: '' });
|
|
expect(commit.mock.calls).toEqual([[types.SEARCH_CONVERSATIONS_SET, []]]);
|
|
});
|
|
|
|
it('sends correct actions if query param is provided and API call is success', async () => {
|
|
axios.get.mockResolvedValue({
|
|
data: {
|
|
payload: [{ messages: [{ id: 1, content: 'value testing' }], id: 1 }],
|
|
},
|
|
});
|
|
|
|
await actions.get({ commit }, { q: 'value' });
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.SEARCH_CONVERSATIONS_SET, []],
|
|
[types.SEARCH_CONVERSATIONS_SET_UI_FLAG, { isFetching: true }],
|
|
[
|
|
types.SEARCH_CONVERSATIONS_SET,
|
|
[{ messages: [{ id: 1, content: 'value testing' }], id: 1 }],
|
|
],
|
|
[types.SEARCH_CONVERSATIONS_SET_UI_FLAG, { isFetching: false }],
|
|
]);
|
|
});
|
|
|
|
it('sends correct actions if query param is provided and API call is errored', async () => {
|
|
axios.get.mockRejectedValue({});
|
|
await actions.get({ commit }, { q: 'value' });
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.SEARCH_CONVERSATIONS_SET, []],
|
|
[types.SEARCH_CONVERSATIONS_SET_UI_FLAG, { isFetching: true }],
|
|
[types.SEARCH_CONVERSATIONS_SET_UI_FLAG, { isFetching: false }],
|
|
]);
|
|
});
|
|
});
|
|
});
|