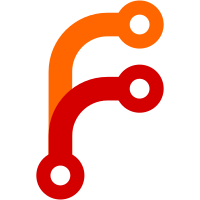
fixes: #3853 - Introduced DISABLE_GRAVATAR Global Config, which will stop chatwoot from making API requests to gravatar - Cleaned up avatar-related logic and centralized it into the avatarable concern - Added specs for the missing cases - Added migration for existing installations to move the avatar to attachment, rather than making the API that results in 404.
36 lines
1 KiB
Ruby
36 lines
1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
module Avatarable
|
|
extend ActiveSupport::Concern
|
|
include Rails.application.routes.url_helpers
|
|
|
|
included do
|
|
has_one_attached :avatar
|
|
validate :acceptable_avatar, if: -> { avatar.changed? }
|
|
after_save :fetch_avatar_from_gravatar
|
|
end
|
|
|
|
def avatar_url
|
|
return url_for(avatar.representation(resize: '250x250')) if avatar.attached? && avatar.representable?
|
|
|
|
''
|
|
end
|
|
|
|
def fetch_avatar_from_gravatar
|
|
return unless saved_changes.key?(:email)
|
|
return if email.blank?
|
|
|
|
# Incase avatar_url is supplied, we don't want to fetch avatar from gravatar
|
|
# So we will wait for it to be processed
|
|
Avatar::AvatarFromGravatarJob.set(wait: 30.seconds).perform_later(self, email)
|
|
end
|
|
|
|
def acceptable_avatar
|
|
return unless avatar.attached?
|
|
|
|
errors.add(:avatar, 'is too big') if avatar.byte_size > 15.megabytes
|
|
|
|
acceptable_types = ['image/jpeg', 'image/png', 'image/gif'].freeze
|
|
errors.add(:avatar, 'filetype not supported') unless acceptable_types.include?(avatar.content_type)
|
|
end
|
|
end
|