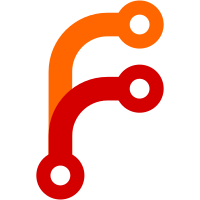
* Use conversationPage module for pagination * Load more conversations * Reset list if conversation status is changed * Add specs to conversationPage * Reset filter when page is re-mounted * Update text * Update text
70 lines
1.5 KiB
JavaScript
70 lines
1.5 KiB
JavaScript
import Vue from 'vue';
|
|
import * as types from '../mutation-types';
|
|
|
|
const state = {
|
|
currentPage: {
|
|
me: 0,
|
|
unassigned: 0,
|
|
all: 0,
|
|
},
|
|
hasEndReached: {
|
|
me: false,
|
|
unassigned: false,
|
|
all: false,
|
|
},
|
|
};
|
|
|
|
export const getters = {
|
|
getHasEndReached: $state => filter => {
|
|
return $state.hasEndReached[filter];
|
|
},
|
|
getCurrentPage: $state => filter => {
|
|
return $state.currentPage[filter];
|
|
},
|
|
};
|
|
|
|
export const actions = {
|
|
setCurrentPage({ commit }, { filter, page }) {
|
|
commit(types.default.SET_CURRENT_PAGE, { filter, page });
|
|
},
|
|
setEndReached({ commit }, { filter }) {
|
|
commit(types.default.SET_CONVERSATION_END_REACHED, { filter });
|
|
},
|
|
reset({ commit }) {
|
|
commit(types.default.CLEAR_CONVERSATION_PAGE);
|
|
},
|
|
};
|
|
|
|
export const mutations = {
|
|
[types.default.SET_CURRENT_PAGE]: ($state, { filter, page }) => {
|
|
Vue.set($state.currentPage, filter, page);
|
|
},
|
|
[types.default.SET_CONVERSATION_END_REACHED]: ($state, { filter }) => {
|
|
if (filter === 'all') {
|
|
Vue.set($state.hasEndReached, 'unassigned', true);
|
|
Vue.set($state.hasEndReached, 'me', true);
|
|
}
|
|
Vue.set($state.hasEndReached, filter, true);
|
|
},
|
|
[types.default.CLEAR_CONVERSATION_PAGE]: $state => {
|
|
$state.currentPage = {
|
|
me: 0,
|
|
unassigned: 0,
|
|
all: 0,
|
|
};
|
|
|
|
$state.hasEndReached = {
|
|
me: false,
|
|
unassigned: false,
|
|
all: false,
|
|
};
|
|
},
|
|
};
|
|
|
|
export default {
|
|
namespaced: true,
|
|
state,
|
|
getters,
|
|
actions,
|
|
mutations,
|
|
};
|