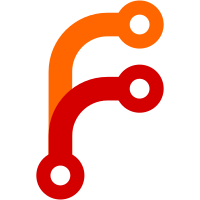
- Initialize an "enterprise" folder that is copyrighted. - You can remove this folder and the system will continue functioning normally, in case you want a purely MIT licensed product. - Enable limit on the number of user accounts in enterprise code. - Use enterprise edition injector methods (inspired from Gitlab). - SaaS software would run enterprise edition software always. Co-authored-by: Pranav Raj S <pranav@chatwoot.com>
37 lines
818 B
Ruby
37 lines
818 B
Ruby
# frozen_string_literal: true
|
|
|
|
module CustomExceptions::Account
|
|
class InvalidEmail < CustomExceptions::Base
|
|
def message
|
|
if @data[:disposable]
|
|
I18n.t 'errors.signup.disposable_email'
|
|
elsif !@data[:valid]
|
|
I18n.t 'errors.signup.invalid_email'
|
|
end
|
|
end
|
|
end
|
|
|
|
class UserExists < CustomExceptions::Base
|
|
def message
|
|
I18n.t('errors.signup.email_already_exists', email: @data[:email])
|
|
end
|
|
end
|
|
|
|
class UserErrors < CustomExceptions::Base
|
|
def message
|
|
@data[:errors].full_messages.join(',')
|
|
end
|
|
end
|
|
|
|
class SignupFailed < CustomExceptions::Base
|
|
def message
|
|
I18n.t 'errors.signup.failed'
|
|
end
|
|
end
|
|
|
|
class PlanUpgradeRequired < CustomExceptions::Base
|
|
def message
|
|
I18n.t 'errors.plan_upgrade_required.failed'
|
|
end
|
|
end
|
|
end
|