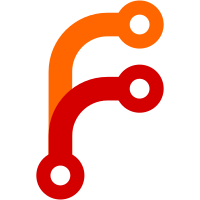
When an agent updates a contact email in the dashboard and the contact also update his email via the email collect box, Merge action receives the same base and mergee contacts which could cause data corruption.
46 lines
1.2 KiB
Ruby
46 lines
1.2 KiB
Ruby
class ContactMergeAction
|
|
pattr_initialize [:account!, :base_contact!, :mergee_contact!]
|
|
|
|
def perform
|
|
# This case happens when an agent updates a contact email in dashboard,
|
|
# while the contact also update his email via email collect box
|
|
return @base_contact if base_contact.id == mergee_contact.id
|
|
|
|
ActiveRecord::Base.transaction do
|
|
validate_contacts
|
|
merge_conversations
|
|
merge_messages
|
|
merge_contact_inboxes
|
|
remove_mergee_contact
|
|
end
|
|
@base_contact
|
|
end
|
|
|
|
private
|
|
|
|
def validate_contacts
|
|
return if belongs_to_account?(@base_contact) && belongs_to_account?(@mergee_contact)
|
|
|
|
raise StandardError, 'contact does not belong to the account'
|
|
end
|
|
|
|
def belongs_to_account?(contact)
|
|
@account.id == contact.account_id
|
|
end
|
|
|
|
def merge_conversations
|
|
Conversation.where(contact_id: @mergee_contact.id).update(contact_id: @base_contact.id)
|
|
end
|
|
|
|
def merge_messages
|
|
Message.where(sender: @mergee_contact).update(sender: @base_contact)
|
|
end
|
|
|
|
def merge_contact_inboxes
|
|
ContactInbox.where(contact_id: @mergee_contact.id).update(contact_id: @base_contact.id)
|
|
end
|
|
|
|
def remove_mergee_contact
|
|
@mergee_contact.destroy!
|
|
end
|
|
end
|