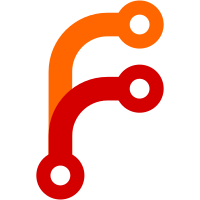
* Add automation modal * Fix the v-model for automations * Actions and Condition dropdowns for automations * Fix merge conflicts * Handle event change and confirmation * Appends new action * Removes actions * Automation api integration * Api integration for creating automations * Registers vuex module to the global store * Automations table * Updarted labels and actions * Integrate automation api * Fixes the mutation error - removed the data key wrapper * Fixed the automation condition models to work with respective event types * Remove temporary fixes added to the api request * Displa timestamp and automation status values * Added the clone buton * Removed uncessary helper method * Specs for automations * Handle WIP code * Remove the payload wrap * Fix the action query payload * Removed unnecessary files * Disabled Automations routes * Ability to delete automations * Fix specs * Fixed merge conflicts Co-authored-by: Fayaz Ahmed <15716057+fayazara@users.noreply.github.com> Co-authored-by: fayazara <fayazara@gmail.com> Co-authored-by: Nithin David Thomas <1277421+nithindavid@users.noreply.github.com> Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com>
94 lines
3.9 KiB
JavaScript
94 lines
3.9 KiB
JavaScript
import axios from 'axios';
|
|
import { actions } from '../../automations';
|
|
import * as types from '../../../mutation-types';
|
|
import automationsList from './fixtures';
|
|
|
|
const commit = jest.fn();
|
|
global.axios = axios;
|
|
jest.mock('axios');
|
|
|
|
describe('#actions', () => {
|
|
describe('#get', () => {
|
|
it('sends correct actions if API is success', async () => {
|
|
axios.get.mockResolvedValue({ data: { payload: automationsList } });
|
|
await actions.get({ commit });
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isFetching: true }],
|
|
[types.default.SET_AUTOMATIONS, automationsList],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isFetching: false }],
|
|
]);
|
|
});
|
|
it('sends correct actions if API is error', async () => {
|
|
axios.get.mockRejectedValue({ message: 'Incorrect header' });
|
|
await actions.get({ commit });
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isFetching: true }],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isFetching: false }],
|
|
]);
|
|
});
|
|
});
|
|
|
|
describe('#create', () => {
|
|
it('sends correct actions if API is success', async () => {
|
|
axios.post.mockResolvedValue({ data: automationsList[0] });
|
|
await actions.create({ commit }, automationsList[0]);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isCreating: true }],
|
|
[types.default.ADD_AUTOMATION, automationsList[0]],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isCreating: false }],
|
|
]);
|
|
});
|
|
it('sends correct actions if API is error', async () => {
|
|
axios.post.mockRejectedValue({ message: 'Incorrect header' });
|
|
await expect(actions.create({ commit })).rejects.toThrow(Error);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isCreating: true }],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isCreating: false }],
|
|
]);
|
|
});
|
|
});
|
|
// API Work in progress
|
|
// describe('#update', () => {
|
|
// it('sends correct actions if API is success', async () => {
|
|
// axios.patch.mockResolvedValue({ data: automationsList[0] });
|
|
// await actions.update({ commit }, automationsList[0]);
|
|
// expect(commit.mock.calls).toEqual([
|
|
// [types.default.SET_AUTOMATION_UI_FLAG, { isUpdating: true }],
|
|
// [types.default.EDIT_AUTOMATION, automationsList[0]],
|
|
// [types.default.SET_AUTOMATION_UI_FLAG, { isUpdating: false }],
|
|
// ]);
|
|
// });
|
|
// it('sends correct actions if API is error', async () => {
|
|
// axios.patch.mockRejectedValue({ message: 'Incorrect header' });
|
|
// await expect(
|
|
// actions.update({ commit }, automationsList[0])
|
|
// ).rejects.toThrow(Error);
|
|
// expect(commit.mock.calls).toEqual([
|
|
// [types.default.SET_AUTOMATION_UI_FLAG, { isUpdating: true }],
|
|
// [types.default.SET_AUTOMATION_UI_FLAG, { isUpdating: false }],
|
|
// ]);
|
|
// });
|
|
// });
|
|
|
|
describe('#delete', () => {
|
|
it('sends correct actions if API is success', async () => {
|
|
axios.delete.mockResolvedValue({ data: automationsList[0] });
|
|
await actions.delete({ commit }, automationsList[0].id);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isDeleting: true }],
|
|
[types.default.DELETE_AUTOMATION, automationsList[0].id],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isDeleting: false }],
|
|
]);
|
|
});
|
|
it('sends correct actions if API is error', async () => {
|
|
axios.delete.mockRejectedValue({ message: 'Incorrect header' });
|
|
await expect(
|
|
actions.delete({ commit }, automationsList[0].id)
|
|
).rejects.toThrow(Error);
|
|
expect(commit.mock.calls).toEqual([
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isDeleting: true }],
|
|
[types.default.SET_AUTOMATION_UI_FLAG, { isDeleting: false }],
|
|
]);
|
|
});
|
|
});
|
|
});
|