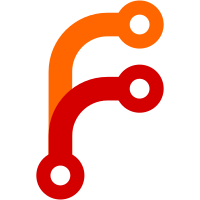
When an agent updates a contact email in the dashboard and the contact also update his email via the email collect box, Merge action receives the same base and mergee contacts which could cause data corruption.
61 lines
2 KiB
Ruby
61 lines
2 KiB
Ruby
require 'rails_helper'
|
|
|
|
describe ::ContactMergeAction do
|
|
subject(:contact_merge) { described_class.new(account: account, base_contact: base_contact, mergee_contact: mergee_contact).perform }
|
|
|
|
let!(:account) { create(:account) }
|
|
let!(:base_contact) { create(:contact, account: account) }
|
|
let!(:mergee_contact) { create(:contact, account: account) }
|
|
|
|
before do
|
|
2.times.each { create(:conversation, contact: base_contact) }
|
|
2.times.each { create(:conversation, contact: mergee_contact) }
|
|
2.times.each { create(:message, sender: mergee_contact) }
|
|
end
|
|
|
|
describe '#perform' do
|
|
it 'deletes mergee_contact' do
|
|
contact_merge
|
|
expect { mergee_contact.reload }.to raise_error(ActiveRecord::RecordNotFound)
|
|
end
|
|
|
|
context 'when base contact and merge contact are same' do
|
|
it 'does not delete contact' do
|
|
mergee_contact = base_contact
|
|
contact_merge
|
|
expect { mergee_contact.reload }.not_to raise_error(ActiveRecord::RecordNotFound)
|
|
end
|
|
end
|
|
|
|
context 'when mergee contact has conversations' do
|
|
it 'moves the conversations to base contact' do
|
|
contact_merge
|
|
expect(base_contact.conversations.count).to be 4
|
|
end
|
|
end
|
|
|
|
context 'when mergee contact has contact inboxes' do
|
|
it 'moves the contact inboxes to base contact' do
|
|
contact_merge
|
|
expect(base_contact.contact_inboxes.count).to be 4
|
|
end
|
|
end
|
|
|
|
context 'when mergee contact has messages' do
|
|
it 'moves the messages to base contact' do
|
|
contact_merge
|
|
expect(base_contact.messages.count).to be 2
|
|
end
|
|
end
|
|
|
|
context 'when contacts belong to a different account' do
|
|
it 'throws an exception' do
|
|
new_account = create(:account)
|
|
expect do
|
|
described_class.new(account: new_account, base_contact: base_contact,
|
|
mergee_contact: mergee_contact).perform
|
|
end.to raise_error('contact does not belong to the account')
|
|
end
|
|
end
|
|
end
|
|
end
|