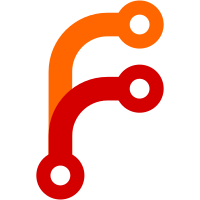
Allow users to mark conversations as unread. Loom video: https://www.loom.com/share/ab70552d3c9c48b685da7dfa64be8bb3 fixes: #5552 Co-authored-by: Pranav Raj S <pranav@chatwoot.com>
35 lines
970 B
JavaScript
35 lines
970 B
JavaScript
import { throwErrorMessage } from 'dashboard/store/utils/api';
|
|
import ConversationApi from '../../../../api/inbox/conversation';
|
|
import mutationTypes from '../../../mutation-types';
|
|
|
|
export default {
|
|
markMessagesRead: async ({ commit }, data) => {
|
|
try {
|
|
const {
|
|
data: { id, agent_last_seen_at: lastSeen },
|
|
} = await ConversationApi.markMessageRead(data);
|
|
setTimeout(
|
|
() =>
|
|
commit(mutationTypes.UPDATE_MESSAGE_UNREAD_COUNT, { id, lastSeen }),
|
|
4000
|
|
);
|
|
} catch (error) {
|
|
// Handle error
|
|
}
|
|
},
|
|
|
|
markMessagesUnread: async ({ commit }, { id }) => {
|
|
try {
|
|
const {
|
|
data: { agent_last_seen_at: lastSeen, unread_count: unreadCount },
|
|
} = await ConversationApi.markMessagesUnread({ id });
|
|
commit(mutationTypes.UPDATE_MESSAGE_UNREAD_COUNT, {
|
|
id,
|
|
lastSeen,
|
|
unreadCount,
|
|
});
|
|
} catch (error) {
|
|
throwErrorMessage(error);
|
|
}
|
|
},
|
|
};
|