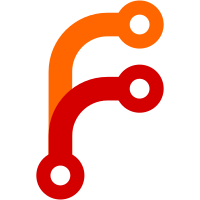
* Added a global config helper to easily access installation/global configs * this will fetch the keys from cache with fallback to DB on cache miss * ability to query multiple keys simultaneously * interface to delete the existing global config cache * Added tests for this new helper module
39 lines
1 KiB
Ruby
39 lines
1 KiB
Ruby
require 'rails_helper'
|
|
|
|
describe GlobalConfig do
|
|
subject(:trigger) { described_class }
|
|
|
|
describe 'execute' do
|
|
context 'when called with default options' do
|
|
before do
|
|
described_class.clear_cache
|
|
end
|
|
|
|
it 'hit DB for the first call' do
|
|
expect(InstallationConfig).to receive(:find_by)
|
|
described_class.get('test')
|
|
end
|
|
|
|
it 'get from cache for subsequent calls' do
|
|
# this loads from DB
|
|
described_class.get('test')
|
|
|
|
# subsequent calls should not hit DB
|
|
expect(InstallationConfig).not_to receive(:find_by)
|
|
described_class.get('test')
|
|
end
|
|
|
|
it 'clears cache and fetch from DB next time, when clear_cache is called' do
|
|
# this loads from DB and is cached
|
|
described_class.get('test')
|
|
|
|
# clears the cache
|
|
described_class.clear_cache
|
|
|
|
# should be loaded from DB
|
|
expect(InstallationConfig).to receive(:find_by).with({ name: 'test' }).and_return(nil)
|
|
described_class.get('test')
|
|
end
|
|
end
|
|
end
|
|
end
|