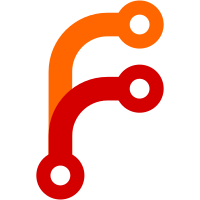
* Ability to change the account name * Ability to set a language to the account Addresses: #667 #307 Co-authored-by: Pranav Raj Sreepuram <pranavrajs@gmail.com>
22 lines
810 B
JavaScript
22 lines
810 B
JavaScript
import queryString from 'query-string';
|
|
|
|
export const frontendURL = (path, params) => {
|
|
const stringifiedParams = params ? `?${queryString.stringify(params)}` : '';
|
|
return `/app/${path}${stringifiedParams}`;
|
|
};
|
|
|
|
export const conversationUrl = (accountId, activeInbox, id) => {
|
|
const path = activeInbox
|
|
? `accounts/${accountId}/inbox/${activeInbox}/conversations/${id}`
|
|
: `accounts/${accountId}/conversations/${id}`;
|
|
return path;
|
|
};
|
|
|
|
export const accountIdFromPathname = pathname => {
|
|
const isInsideAccountScopedURLs = pathname.includes('/app/accounts');
|
|
const urlParam = pathname.split('/')[3];
|
|
// eslint-disable-next-line no-restricted-globals
|
|
const isScoped = isInsideAccountScopedURLs && !isNaN(urlParam);
|
|
const accountId = isScoped ? Number(urlParam) : '';
|
|
return accountId;
|
|
};
|