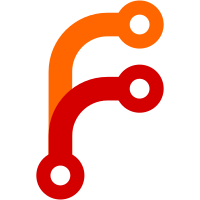
* Changes for the message to have multiple attachments * changed the message association to attachments from has_one to has_many * changed all the references of this association in building and fetching to reflect this change * Added number of attachments validation to the message model * Modified the backend responses and endpoints to reflect multiple attachment support (#737) * Changing the frontend components for multiple attachments * changed the request structure to reflect the multiple attachment structures * changed the message bubbles to support multiple attachments * bugfix: agent side attachment was not showing because of a missing await * broken message was shown because of the store filtering * Added documentation for ImageMagick * spec fixes * refactored code to reflect more apt namings * Added updated message listener for the dashboard (#727) * Added the publishing for message updated event * Implemented the listener for dashboard Co-authored-by: Pranav Raj Sreepuram <pranavrajs@gmail.com>
133 lines
3.1 KiB
Vue
133 lines
3.1 KiB
Vue
<template>
|
|
<div
|
|
class="conversation"
|
|
:class="{ active: isActiveChat, 'unread-chat': hasUnread }"
|
|
@click="cardClick(chat)"
|
|
>
|
|
<Thumbnail
|
|
v-if="!hideThumbnail"
|
|
:src="chat.meta.sender.thumbnail"
|
|
:badge="chat.meta.sender.channel"
|
|
class="columns"
|
|
:username="chat.meta.sender.name"
|
|
size="40px"
|
|
/>
|
|
<div class="conversation--details columns">
|
|
<h4 class="conversation--user">
|
|
{{ chat.meta.sender.name }}
|
|
<span
|
|
v-if="!hideInboxName && isInboxNameVisible"
|
|
v-tooltip.bottom="inboxName(chat.inbox_id)"
|
|
class="label"
|
|
>
|
|
{{ inboxName(chat.inbox_id) }}
|
|
</span>
|
|
</h4>
|
|
<p
|
|
class="conversation--message"
|
|
v-html="extractMessageText(lastMessage(chat))"
|
|
></p>
|
|
|
|
<div class="conversation--meta">
|
|
<span class="timestamp">
|
|
{{ dynamicTime(lastMessage(chat).created_at) }}
|
|
</span>
|
|
<span class="unread">{{ getUnreadCount }}</span>
|
|
</div>
|
|
</div>
|
|
</div>
|
|
</template>
|
|
<script>
|
|
/* eslint no-console: 0 */
|
|
/* eslint no-extra-boolean-cast: 0 */
|
|
import { mapGetters } from 'vuex';
|
|
import Thumbnail from '../Thumbnail';
|
|
import getEmojiSVG from '../emoji/utils';
|
|
import conversationMixin from '../../../mixins/conversations';
|
|
import timeMixin from '../../../mixins/time';
|
|
import router from '../../../routes';
|
|
import { frontendURL, conversationUrl } from '../../../helper/URLHelper';
|
|
|
|
export default {
|
|
components: {
|
|
Thumbnail,
|
|
},
|
|
|
|
mixins: [timeMixin, conversationMixin],
|
|
props: {
|
|
chat: {
|
|
type: Object,
|
|
default: () => {},
|
|
},
|
|
hideInboxName: {
|
|
type: Boolean,
|
|
default: false,
|
|
},
|
|
hideThumbnail: {
|
|
type: Boolean,
|
|
default: false,
|
|
},
|
|
},
|
|
|
|
computed: {
|
|
...mapGetters({
|
|
currentChat: 'getSelectedChat',
|
|
inboxesList: 'inboxes/getInboxes',
|
|
activeInbox: 'getSelectedInbox',
|
|
currentUser: 'getCurrentUser',
|
|
}),
|
|
|
|
isActiveChat() {
|
|
return this.currentChat.id === this.chat.id;
|
|
},
|
|
|
|
getUnreadCount() {
|
|
return this.unreadMessagesCount(this.chat);
|
|
},
|
|
|
|
hasUnread() {
|
|
return this.getUnreadCount > 0;
|
|
},
|
|
|
|
isInboxNameVisible() {
|
|
return !this.activeInbox;
|
|
},
|
|
},
|
|
|
|
methods: {
|
|
cardClick(chat) {
|
|
const { activeInbox } = this;
|
|
const path = conversationUrl(
|
|
this.currentUser.account_id,
|
|
activeInbox,
|
|
chat.id
|
|
);
|
|
router.push({ path: frontendURL(path) });
|
|
},
|
|
extractMessageText(chatItem) {
|
|
const { content, attachments } = chatItem;
|
|
|
|
if (content) {
|
|
return content;
|
|
}
|
|
if (!attachments) {
|
|
return ' ';
|
|
}
|
|
|
|
const [attachment] = attachments;
|
|
const { file_type: fileType } = attachment;
|
|
const key = `CHAT_LIST.ATTACHMENTS.${fileType}`;
|
|
return `
|
|
<i class="small-icon ${this.$t(`${key}.ICON`)}"></i>
|
|
${this.$t(`${key}.CONTENT`)}
|
|
`;
|
|
},
|
|
getEmojiSVG,
|
|
|
|
inboxName(inboxId) {
|
|
const stateInbox = this.$store.getters['inboxes/getInbox'](inboxId);
|
|
return stateInbox.name || '';
|
|
},
|
|
},
|
|
};
|
|
</script>
|