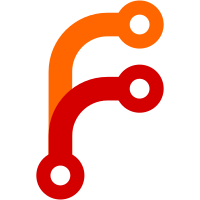
When sending the message with audio, only the signed id of the file is sent. In the back end check only the UploadedFile type. The attachment has the default file type image, now it gets the content type from the signed id Fixes: #5375 Co-authored-by: Sojan Jose <sojan@pepalo.com>
106 lines
3.2 KiB
Ruby
106 lines
3.2 KiB
Ruby
class Messages::MessageBuilder
|
|
include ::FileTypeHelper
|
|
attr_reader :message
|
|
|
|
def initialize(user, conversation, params)
|
|
@params = params
|
|
@private = params[:private] || false
|
|
@conversation = conversation
|
|
@user = user
|
|
@message_type = params[:message_type] || 'outgoing'
|
|
@attachments = params[:attachments]
|
|
@automation_rule = @params&.dig(:content_attributes, :automation_rule_id)
|
|
return unless params.instance_of?(ActionController::Parameters)
|
|
|
|
@in_reply_to = params.to_unsafe_h&.dig(:content_attributes, :in_reply_to)
|
|
@items = params.to_unsafe_h&.dig(:content_attributes, :items)
|
|
end
|
|
|
|
def perform
|
|
@message = @conversation.messages.build(message_params)
|
|
process_attachments
|
|
process_emails
|
|
@message.save!
|
|
@message
|
|
end
|
|
|
|
private
|
|
|
|
def process_attachments
|
|
return if @attachments.blank?
|
|
|
|
@attachments.each do |uploaded_attachment|
|
|
attachment = @message.attachments.build(
|
|
account_id: @message.account_id,
|
|
file: uploaded_attachment
|
|
)
|
|
|
|
attachment.file_type = if uploaded_attachment.is_a?(String)
|
|
file_type_by_signed_id(
|
|
uploaded_attachment
|
|
)
|
|
else
|
|
file_type(uploaded_attachment&.content_type)
|
|
end
|
|
end
|
|
end
|
|
|
|
def process_emails
|
|
return unless @conversation.inbox&.inbox_type == 'Email'
|
|
|
|
cc_emails = @params[:cc_emails].split(',') if @params[:cc_emails]
|
|
bcc_emails = @params[:bcc_emails].split(',') if @params[:bcc_emails]
|
|
|
|
@message.content_attributes[:cc_emails] = cc_emails
|
|
@message.content_attributes[:bcc_emails] = bcc_emails
|
|
end
|
|
|
|
def message_type
|
|
if @conversation.inbox.channel_type != 'Channel::Api' && @message_type == 'incoming'
|
|
raise StandardError, 'Incoming messages are only allowed in Api inboxes'
|
|
end
|
|
|
|
@message_type
|
|
end
|
|
|
|
def sender
|
|
message_type == 'outgoing' ? (message_sender || @user) : @conversation.contact
|
|
end
|
|
|
|
def external_created_at
|
|
@params[:external_created_at].present? ? { external_created_at: @params[:external_created_at] } : {}
|
|
end
|
|
|
|
def automation_rule_id
|
|
@automation_rule.present? ? { content_attributes: { automation_rule_id: @automation_rule } } : {}
|
|
end
|
|
|
|
def campaign_id
|
|
@params[:campaign_id].present? ? { additional_attributes: { campaign_id: @params[:campaign_id] } } : {}
|
|
end
|
|
|
|
def template_params
|
|
@params[:template_params].present? ? { additional_attributes: { template_params: JSON.parse(@params[:template_params].to_json) } } : {}
|
|
end
|
|
|
|
def message_sender
|
|
return if @params[:sender_type] != 'AgentBot'
|
|
|
|
AgentBot.where(account_id: [nil, @conversation.account.id]).find_by(id: @params[:sender_id])
|
|
end
|
|
|
|
def message_params
|
|
{
|
|
account_id: @conversation.account_id,
|
|
inbox_id: @conversation.inbox_id,
|
|
message_type: message_type,
|
|
content: @params[:content],
|
|
private: @private,
|
|
sender: sender,
|
|
content_type: @params[:content_type],
|
|
items: @items,
|
|
in_reply_to: @in_reply_to,
|
|
echo_id: @params[:echo_id]
|
|
}.merge(external_created_at).merge(automation_rule_id).merge(campaign_id).merge(template_params)
|
|
end
|
|
end
|