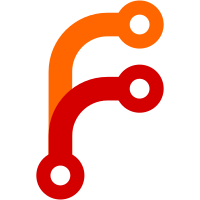
* Add automation modal * Fix the v-model for automations * Actions and Condition dropdowns for automations * Fix merge conflicts * Handle event change and confirmation * Appends new action * Removes actions * Automation api integration * Api integration for creating automations * Registers vuex module to the global store * Automations table * Updarted labels and actions * Integrate automation api * Fixes the mutation error - removed the data key wrapper * Fixed the automation condition models to work with respective event types * Remove temporary fixes added to the api request * Displa timestamp and automation status values * Added the clone buton * Removed uncessary helper method * Specs for automations * Handle WIP code * Remove the payload wrap * Fix the action query payload * Removed unnecessary files * Disabled Automations routes * Ability to delete automations * Fix specs * Fixed merge conflicts Co-authored-by: Fayaz Ahmed <15716057+fayazara@users.noreply.github.com> Co-authored-by: fayazara <fayazara@gmail.com> Co-authored-by: Nithin David Thomas <1277421+nithindavid@users.noreply.github.com> Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com>
41 lines
900 B
JavaScript
41 lines
900 B
JavaScript
import actionQueryGenerator from '../actionQueryGenerator';
|
|
|
|
const testData = [
|
|
{
|
|
action_name: 'add_label',
|
|
action_params: [{ id: 'testlabel', name: 'testlabel' }],
|
|
},
|
|
{
|
|
action_name: 'assign_team',
|
|
action_params: [
|
|
{
|
|
id: 1,
|
|
name: 'sales team',
|
|
description: 'This is our internal sales team',
|
|
allow_auto_assign: true,
|
|
account_id: 1,
|
|
is_member: true,
|
|
},
|
|
],
|
|
},
|
|
];
|
|
|
|
const finalResult = [
|
|
{
|
|
action_name: 'add_label',
|
|
action_params: ['testlabel'],
|
|
},
|
|
{
|
|
action_name: 'assign_team',
|
|
action_params: [1],
|
|
},
|
|
];
|
|
|
|
describe('#actionQueryGenerator', () => {
|
|
it('returns the correct format of filter query', () => {
|
|
expect(actionQueryGenerator(testData)).toEqual(finalResult);
|
|
expect(
|
|
actionQueryGenerator(testData).every(i => Array.isArray(i.action_params))
|
|
).toBe(true);
|
|
});
|
|
});
|