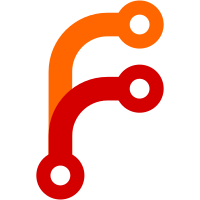
* Add automation modal * Fix the v-model for automations * Actions and Condition dropdowns for automations * Fix merge conflicts * Handle event change and confirmation * Appends new action * Removes actions * Automation api integration * Api integration for creating automations * Registers vuex module to the global store * Automations table * Updarted labels and actions * Integrate automation api * Fixes the mutation error - removed the data key wrapper * Fixed the automation condition models to work with respective event types * Remove temporary fixes added to the api request * Displa timestamp and automation status values * Added the clone buton * Removed uncessary helper method * Specs for automations * Handle WIP code * Remove the payload wrap * Fix the action query payload * Removed unnecessary files * Disabled Automations routes * Ability to delete automations * Fix specs * Edit automation modal * Edit automation modal and api integration * Replaced hardcoded values * Using absolute paths * Update app/javascript/dashboard/routes/dashboard/settings/automation/EditAutomationRule.vue Co-authored-by: Nithin David Thomas <1277421+nithindavid@users.noreply.github.com> * Update app/javascript/dashboard/routes/dashboard/settings/automation/Index.vue Co-authored-by: Nithin David Thomas <1277421+nithindavid@users.noreply.github.com> * Intendation fix * Disable automation route * Minor fix Co-authored-by: Muhsin Keloth <muhsinkeramam@gmail.com> Co-authored-by: Nithin David Thomas <1277421+nithindavid@users.noreply.github.com> Co-authored-by: Sivin Varghese <64252451+iamsivin@users.noreply.github.com>
112 lines
3 KiB
JavaScript
112 lines
3 KiB
JavaScript
import { frontendURL } from '../../../../helper/URLHelper';
|
|
|
|
const settings = accountId => ({
|
|
parentNav: 'settings',
|
|
routes: [
|
|
'agent_list',
|
|
'canned_list',
|
|
'labels_list',
|
|
'settings_inbox',
|
|
'attributes_list',
|
|
'settings_inbox_new',
|
|
'settings_inbox_list',
|
|
'settings_inbox_show',
|
|
'settings_inboxes_page_channel',
|
|
'settings_inboxes_add_agents',
|
|
'settings_inbox_finish',
|
|
'settings_integrations',
|
|
'settings_integrations_webhook',
|
|
'settings_integrations_integration',
|
|
'settings_applications',
|
|
'settings_applications_webhook',
|
|
'settings_applications_integration',
|
|
'general_settings',
|
|
'general_settings_index',
|
|
'settings_teams_list',
|
|
'settings_teams_new',
|
|
'settings_teams_add_agents',
|
|
'settings_teams_finish',
|
|
'settings_teams_edit',
|
|
'settings_teams_edit_members',
|
|
'settings_teams_edit_finish',
|
|
'automation_list',
|
|
],
|
|
menuItems: [
|
|
{
|
|
icon: 'people',
|
|
label: 'AGENTS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/agents/list`),
|
|
toStateName: 'agent_list',
|
|
},
|
|
{
|
|
icon: 'people-team',
|
|
label: 'TEAMS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/teams/list`),
|
|
toStateName: 'settings_teams_list',
|
|
},
|
|
{
|
|
icon: 'mail-inbox-all',
|
|
label: 'INBOXES',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/inboxes/list`),
|
|
toStateName: 'settings_inbox_list',
|
|
},
|
|
{
|
|
icon: 'tag',
|
|
label: 'LABELS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/labels/list`),
|
|
toStateName: 'labels_list',
|
|
},
|
|
{
|
|
icon: 'code',
|
|
label: 'CUSTOM_ATTRIBUTES',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(
|
|
`accounts/${accountId}/settings/custom-attributes/list`
|
|
),
|
|
toStateName: 'attributes_list',
|
|
},
|
|
// {
|
|
// icon: 'automation',
|
|
// label: 'AUTOMATION',
|
|
// hasSubMenu: false,
|
|
// toState: frontendURL(`accounts/${accountId}/settings/automation/list`),
|
|
// toStateName: 'automation_list',
|
|
// },
|
|
{
|
|
icon: 'chat-multiple',
|
|
label: 'CANNED_RESPONSES',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(
|
|
`accounts/${accountId}/settings/canned-response/list`
|
|
),
|
|
toStateName: 'canned_list',
|
|
},
|
|
{
|
|
icon: 'flash-on',
|
|
label: 'INTEGRATIONS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/integrations`),
|
|
toStateName: 'settings_integrations',
|
|
},
|
|
{
|
|
icon: 'star-emphasis',
|
|
label: 'APPLICATIONS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/applications`),
|
|
toStateName: 'settings_applications',
|
|
},
|
|
{
|
|
icon: 'settings',
|
|
label: 'ACCOUNT_SETTINGS',
|
|
hasSubMenu: false,
|
|
toState: frontendURL(`accounts/${accountId}/settings/general`),
|
|
toStateName: 'general_settings_index',
|
|
},
|
|
],
|
|
});
|
|
|
|
export default settings;
|