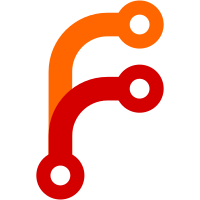
* Feature: Move to the next conversation when I resolve a the current conversation * check if nextId is present before emitting the event * use es6 string literals * use a named variable for better reading * create a variable for better readability * better sintax to get clickable element * after last, go to first chat when resolve * use state and action to set next chat * remove not used emit * clear selected state when there is not next chat * Remove deprecated scope from FB Channel (#761) Remove deprecated scope from FB Channel * Feature: Customise the position of messenger (#767) Co-authored-by: Nithin David Thomas <webofnithin@gmail.com> * Bug: Redirect user to set new password screen (#772) * auto linter * fix js linter * sort chats on getter / filter before getting next chat * Revert not related changes on ConversationCard.vue * add test for getNextChatConversation getter * remove not used module * add test for getAllConversations getter
69 lines
2.2 KiB
JavaScript
69 lines
2.2 KiB
JavaScript
import authAPI from '../../../api/auth';
|
|
|
|
export const getSelectedChatConversation = ({
|
|
allConversations,
|
|
selectedChat,
|
|
}) =>
|
|
allConversations.filter(conversation => conversation.id === selectedChat.id);
|
|
|
|
// getters
|
|
const getters = {
|
|
getAllConversations: ({ allConversations }) => allConversations.sort(
|
|
(a, b) => b.messages.last().created_at - a.messages.last().created_at
|
|
),
|
|
getSelectedChat: ({ selectedChat }) => selectedChat,
|
|
getMineChats(_state) {
|
|
const currentUserID = authAPI.getCurrentUser().id;
|
|
return _state.allConversations.filter(chat =>
|
|
chat.meta.assignee === null
|
|
? false
|
|
: chat.status === _state.chatStatusFilter &&
|
|
chat.meta.assignee.id === currentUserID
|
|
);
|
|
},
|
|
getUnAssignedChats(_state) {
|
|
return _state.allConversations.filter(
|
|
chat =>
|
|
chat.meta.assignee === null && chat.status === _state.chatStatusFilter
|
|
);
|
|
},
|
|
getAllStatusChats(_state) {
|
|
return _state.allConversations.filter(
|
|
chat => chat.status === _state.chatStatusFilter
|
|
);
|
|
},
|
|
getChatListLoadingStatus: ({ listLoadingStatus }) => listLoadingStatus,
|
|
getAllMessagesLoaded(_state) {
|
|
const [chat] = getSelectedChatConversation(_state);
|
|
return !chat || chat.allMessagesLoaded === undefined
|
|
? false
|
|
: chat.allMessagesLoaded;
|
|
},
|
|
getUnreadCount(_state) {
|
|
const [chat] = getSelectedChatConversation(_state);
|
|
if (!chat) return [];
|
|
return chat.messages.filter(
|
|
chatMessage =>
|
|
chatMessage.created_at * 1000 > chat.agent_last_seen_at * 1000 &&
|
|
chatMessage.message_type === 0 &&
|
|
chatMessage.private !== true
|
|
).length;
|
|
},
|
|
getChatStatusFilter: ({ chatStatusFilter }) => chatStatusFilter,
|
|
getSelectedInbox: ({ currentInbox }) => currentInbox,
|
|
getConvTabStats: ({ convTabStats }) => convTabStats,
|
|
getNextChatConversation: (_state) => {
|
|
const { selectedChat } = _state;
|
|
const conversations = getters.getAllStatusChats(_state);
|
|
if (conversations.length <= 1) {
|
|
return null;
|
|
};
|
|
const currentIndex = conversations.findIndex(
|
|
conversation => conversation.id === selectedChat.id
|
|
);
|
|
const nextIndex = (currentIndex + 1) % conversations.length;
|
|
return conversations[nextIndex];
|
|
},
|
|
};
|
|
|
|
export default getters;
|